030 Introducing NumPy#
COM6018
Copyright © 2023, 2024 Jon Barker, University of Sheffield. All rights reserved.
In this lab class we are going to use NumPy to analyse the atmospheric gas concentration data that we saw in the previous lab. We will start by performing some simple tasks without using NumPy, and then we will see how NumPy can make our lives easier.
Step 1 - Reading the data#
Use ideas from the previous lab class to read the file data/co2e.csv
into a variable called co2e_data
that represents the file contents as a list of dictionaries. Make sure that the dictionary entries are of the correct type (i.e., the year
entry should be an integer, and the co2_concentration
entry should be a float).
Write your solution in the cell below and then run the test cell to check your solution.
# SOLUTION
import csv
def read_csv(filename):
with open(filename, 'r') as fp:
reader = csv.DictReader(fp, skipinitialspace=True, delimiter=',')
data = [row for row in reader]
return data
def fix_data_types(data, fields):
for row in data:
for k in fields:
row[k] = fields[k](row[k])
return data
co2e_data = read_csv('data/co2e.csv')
co2e_data = fix_data_types(co2e_data, {'year': int, 'month':int, 'day': int, 'co2_concentration': float, 'ch4_concentration': float, 'co2e': float, 'decimal_year': float})
print(co2e_data[0])
{'year': 1987, 'month': 4, 'day': 3, 'co2_concentration': 350.84, 'ch4_concentration': 1.70002, 'co2e': 393.34049999999996, 'decimal_year': 1987.2554794520547}
# TEST
assert (co2e_data[0]) == {'year': 1987,
'month': 4,
'day': 3,
'co2_concentration': 350.84,
'ch4_concentration': 1.70002,
'co2e': 393.34049999999996,
'decimal_year': 1987.2554794520547}
print('All tests passed!')
All tests passed!
Step 2 - Extracting the data#
We want to analyse the atmospheric gas concentrations as a sequence of values that change over time. The way that the data is currently stored does not make this very easy. We need to extract the data from each field in the list of dictionaries and store it in a more convenient form, such as a list. For example, we would like all of the co2_concentration
values to be stored in a list called co2_concentrations
.
Write a function called extract_data
that takes the list of dictionaries and a field name as input and returns a list of values for that field. For example, extract_data(co2e_data, 'co2_concentration')
should return a list of CO2 concentrations.
# SOLUTION
def extract_data(data, field):
return [entry[field] for entry in data]
co2_concentration = extract_data(co2e_data, 'co2_concentration')
print(co2_concentration)
[350.84, 350.28, 350.4, 349.47, 349.81, 350.01, 350.13, 350.56, 350.33, 351.33, 351.18, 351.23, 351.1, 351.72, 351.58, 351.21, 351.23, 351.33, 351.02, 351.23, 351.61, 351.68, 351.04, 350.95, 352.38, 352.16, 352.27, 352.12, 352.11, 351.9, 351.92, 351.8, 351.56, 351.51, 351.75, 351.83, 351.72, 351.52, 351.4, 351.8, 352.04, 351.44, 351.6, 351.71, 352.13, 352.04, 351.88, 352.13, 352.04, 352.06, 351.25, 351.77, 351.24, 351.41, 351.62, 351.45, 351.4, 351.42, 351.13, 351.03, 350.75, 350.78, 350.95, 351.09, 351.1, 351.13, 350.69, 350.83, 351.09, 350.81, 351.1, 351.22, 350.81, 350.68, 349.79, 349.57, 348.97, 350.5, 350.67, 350.57, 350.56, 350.39, 350.4, 347.64, 349.66, 347.8, 349.07, 349.22, 349.89, 350.09, 348.15, 347.82, 348.07, 348.01, 348.14, 345.97, 346.23, 346.65, 346.25, 346.67, 346.95, 346.62, 346.89, 346.12, 346.38, 346.99, 346.91, 346.8, 346.89, 347.03, 344.97, 344.74, 344.64, 345.39, 346.06, 346.29, 346.11, 345.52, 345.4, 345.25, 346.51, 346.53, 345.8, 345.64, 345.17, 346.4, 345.96, 346.08, 347.01, 346.99, 346.58, 346.62, 346.49, 346.22, 346.34, 346.37, 346.02, 346.05, 346.98, 346.85, 346.89, 346.94, 347.5, 347.14, 347.21, 347.16, 347.18, 347.32, 347.88, 347.7, 347.63, 347.93, 348.58, 348.22, 348.08, 348.63, 348.09, 348.19, 348.93, 348.84, 348.84, 348.97, 348.84, 348.91, 348.73, 348.72, 348.9, 349.13, 349.06, 348.95, 349.25, 348.85, 349.53, 349.79, 350.22, 350.01, 349.86, 350.59, 350.68, 350.21, 350.71, 350.68, 350.74, 350.77, 351.71, 351.22, 351.11, 350.91, 351.02, 350.87, 350.94, 351.45, 351.32, 351.24, 351.22, 350.79, 350.93, 351.57, 351.63, 351.85, 350.81, 351.02, 352.39, 352.32, 351.93, 353.4, 352.92, 352.27, 352.5, 352.43, 352.21, 351.76, 350.98, 350.89, 351.11, 351.92, 351.04, 350.94, 351.96, 352.06, 352.81, 352.99, 352.9, 353.22, 353.08, 353.72, 354.03, 353.37, 353.3, 353.0, 352.63, 352.62, 353.18, 353.64, 353.75, 353.68, 353.5, 353.68, 354.12, 354.3, 353.29, 353.26, 353.04, 353.42, 353.88, 353.92, 354.0, 354.08, 353.98, 352.99, 353.97, 353.95, 353.64, 354.48, 354.33, 354.49, 354.5, 354.32, 354.24, 354.02, 353.43, 353.73, 353.71, 353.78, 354.07, 354.02, 354.14, 354.25, 354.43, 354.08, 353.79, 353.69, 353.68, 353.9, 353.36, 353.37, 353.42, 353.12, 353.06, 353.14, 352.91, 352.91, 352.95, 352.88, 352.91, 353.03, 352.87, 352.81, 352.88, 352.86, 352.68, 352.3, 352.3, 352.0, 352.9, 352.58, 352.23, 352.06, 351.72, 351.73, 351.25, 351.44, 352.27, 352.23, 351.29, 350.86, 350.95, 350.86, 350.4, 350.64, 350.61, 350.36, 350.45, 350.27, 349.17, 349.42, 350.4, 349.66, 350.15, 350.81, 348.5, 348.69, 349.54, 349.28, 348.36, 348.56, 348.47, 348.53, 348.51, 349.14, 349.19, 348.07, 347.93, 347.58, 348.12, 347.98, 348.62, 347.91, 348.79, 348.63, 348.4, 348.84, 348.43, 348.15, 348.14, 347.74, 348.37, 348.61, 349.18, 349.1, 349.16, 348.74, 349.37, 349.09, 349.06, 349.07, 349.73, 349.35, 349.41, 349.57, 349.48, 349.68, 349.89, 349.76, 349.81, 349.73, 349.68, 349.9, 350.03, 349.82, 350.46, 350.05, 350.31, 350.23, 350.33, 350.35, 350.02, 349.92, 350.31, 350.53, 350.46, 350.54, 350.59, 351.15, 351.19, 351.37, 351.4, 351.96, 351.33, 351.08, 351.12, 351.11, 351.28, 352.17, 352.26, 352.85, 352.64, 351.71, 351.84, 352.12, 352.22, 352.09, 352.51, 352.89, 352.7, 353.02, 352.83, 352.58, 352.31, 352.69, 353.25, 352.82, 353.1, 352.4, 352.52, 352.42, 352.29, 352.05, 352.04, 352.14, 352.91, 352.61, 352.82, 352.91, 353.69, 353.09, 352.96, 353.18, 352.71, 352.46, 352.34, 352.08, 352.17, 353.57, 353.06, 352.94, 352.91, 352.92, 352.93, 353.44, 353.51, 353.35, 353.9, 353.69, 353.36, 353.3, 353.34, 353.28, 352.52, 352.19, 352.65, 353.57, 354.78, 352.73, 353.44, 353.56, 353.22, 353.06, 352.78, 352.92, 352.35, 352.77, 352.94, 354.07, 354.09, 354.37, 353.92, 353.95, 354.83, 354.32, 354.19, 353.88, 354.07, 354.29, 354.65, 354.44, 354.3, 354.33, 353.75, 353.72, 355.56, 355.42, 355.23, 355.33, 354.34, 354.58, 355.3, 355.88, 355.52, 354.81, 355.35, 355.24, 355.75, 355.79, 356.23, 356.25, 355.69, 355.61, 355.62, 355.51, 356.17, 356.45, 355.61, 355.0, 355.2, 355.09, 355.34, 355.28, 355.57, 355.65, 356.06, 355.98, 355.42, 355.69, 355.54, 355.47, 355.17, 355.25, 355.22, 355.24, 356.27, 355.74, 355.69, 355.57, 355.68, 355.74, 355.48, 355.43, 353.69, 354.74, 354.5, 355.83, 354.76, 354.63, 354.85, 353.72, 353.51, 353.7, 353.59, 354.74, 354.81, 354.39, 354.52, 354.37, 353.39, 353.43, 353.45, 353.9, 353.61, 352.85, 352.81, 352.79, 352.93, 353.07, 352.73, 352.75, 352.54, 351.86, 350.78, 351.48, 351.21, 350.74, 350.59, 350.57, 350.78, 350.1, 349.2, 349.49, 348.36, 348.97, 349.01, 348.89, 349.43, 349.1, 349.2, 348.92, 348.96, 348.91, 350.44, 350.08, 350.24, 349.4, 349.42, 350.06, 349.47, 349.25, 350.14, 350.41, 350.22, 349.9, 349.95, 349.36, 350.19, 350.36, 350.3, 350.09, 349.93, 350.31, 350.48, 350.12, 349.86, 350.72, 350.85, 350.65, 350.9, 351.29, 350.99, 351.17, 351.13, 351.34, 351.46, 351.7, 351.91, 351.48, 351.36, 351.75, 351.8, 351.41, 351.11, 351.15, 351.23, 351.68, 352.32, 351.99, 351.98, 352.07, 352.17, 351.87, 351.82, 352.07, 353.99, 353.6, 352.11, 351.85, 353.2, 352.32, 352.81, 352.97, 352.29, 352.36, 352.89, 352.59, 352.11, 352.11, 352.22, 353.32, 353.53, 353.44, 353.43, 353.17, 353.22, 353.29, 353.15, 353.14, 353.1, 353.27, 353.91, 353.39, 353.04, 353.09, 353.26, 353.55, 353.73, 353.85, 353.55, 353.54, 353.55, 353.79, 353.98, 354.05, 354.23, 354.12, 353.68, 353.47, 355.83, 355.34, 354.35, 354.64, 355.34, 355.6, 354.42, 353.89, 354.1, 354.1, 355.68, 355.22, 355.0, 354.91, 354.93, 354.67, 354.27, 354.01, 354.5, 354.61, 355.44, 355.81, 355.6, 355.87, 355.33, 355.54, 354.96, 354.88, 354.95, 354.93, 354.57, 356.53, 356.34, 355.13, 355.29, 355.54, 355.98, 355.37, 355.35, 356.63, 356.24, 356.04, 355.57, 355.99, 356.01, 355.59, 355.77, 355.84, 356.1, 355.41, 354.88, 356.3, 356.31, 356.5, 356.74, 356.07, 355.52, 355.5, 355.62, 355.75, 356.24, 356.86, 356.17, 357.3, 357.46, 357.51, 357.38, 356.98, 356.9, 357.02, 356.81, 356.63, 356.71, 356.63, 356.69, 357.03, 356.91, 356.81, 356.64, 357.0, 356.88, 357.15, 356.98, 356.98, 356.89, 356.98, 356.91, 356.53, 357.18, 357.08, 356.32, 356.36, 356.58, 356.69, 356.58, 356.32, 356.13, 356.15, 356.49, 355.83, 355.03, 355.58, 355.93, 355.72, 355.47, 355.54, 355.37, 355.39, 355.3, 355.44, 356.02, 355.69, 355.93, 355.73, 355.7, 355.29, 354.98, 354.56, 355.1, 355.17, 355.24, 354.08, 353.55, 352.92, 352.59, 353.86, 353.81, 354.5, 354.71, 354.93, 354.92, 354.55, 354.58, 354.01, 353.62, 353.36, 353.31, 353.61, 353.73, 353.82, 352.73, 353.22, 352.14, 352.35, 352.67, 352.59, 350.49, 351.16, 351.01, 351.2, 350.36, 350.81, 351.11, 351.05, 350.3, 350.96, 349.16, 350.99, 351.25, 351.32, 351.34, 351.12, 351.0, 350.26, 350.61, 350.65, 350.84, 350.77, 350.57, 350.91, 351.05, 350.9, 350.79, 350.98, 351.06, 350.6, 351.48, 351.38, 351.21, 351.13, 351.21, 351.69, 351.84, 351.94, 352.11, 352.63, 352.27, 352.12, 352.18, 352.63, 352.65, 352.87, 352.61, 352.88, 353.4, 352.93, 352.94, 352.85, 352.97, 352.94, 353.0, 353.03, 353.06, 353.17, 353.13, 353.3, 353.68, 353.7, 353.55, 353.36, 353.43, 353.25, 353.45, 354.73, 354.36, 353.8, 353.75, 353.57, 353.51, 353.89, 353.8, 353.87, 354.13, 354.54, 354.35, 354.15, 354.02, 354.15, 354.62, 354.37, 354.25, 355.36, 354.74, 354.68, 355.7, 354.71, 354.33, 354.04, 354.01, 354.11, 354.12, 353.98, 354.07, 354.36, 354.8, 354.9, 354.19, 354.61, 354.83, 354.81, 355.87, 355.89, 354.61, 353.83, 354.5, 354.39, 354.1, 355.42, 355.15, 355.07, 355.55, 355.37, 355.34, 354.62, 354.56, 354.91, 355.26, 355.14, 354.98, 355.28, 354.88, 354.97, 354.91, 356.03, 356.1, 356.29, 356.81, 356.85, 355.78, 355.81, 355.96, 354.88, 355.71, 355.4, 355.46, 355.65, 354.92, 355.35, 357.52, 357.27, 356.15, 356.99, 356.9, 356.58, 357.05, 357.32, 357.32, 357.03, 356.95, 357.63, 357.19, 357.47, 358.61, 357.95, 357.6, 358.39, 358.49, 358.15, 357.09, 357.49, 357.14, 357.46, 357.59, 358.16, 358.32, 358.32, 359.42, 359.4, 358.9, 358.78, 358.99, 359.08, 359.13, 358.86, 358.6, 358.83, 358.91, 359.03, 358.6, 358.92, 358.39, 359.38, 359.93, 359.91, 359.71, 359.49, 358.74, 358.46, 358.28, 358.29, 358.79, 358.55, 358.01, 357.86, 357.95, 357.97, 358.43, 358.14, 358.3, 358.2, 358.34, 357.88, 358.0, 358.15, 358.49, 357.87, 358.08, 357.87, 357.92, 357.76, 357.84, 357.65, 357.97, 357.93, 357.97, 357.86, 357.86, 357.74, 357.55, 356.1, 357.74, 357.84, 356.84, 356.86, 356.79, 356.15, 356.21, 356.35, 356.4, 355.37, 354.78, 354.89, 350.92, 355.42, 355.0, 355.23, 355.41, 355.25, 354.44, 354.12, 354.54, 354.85, 354.37, 353.34, 352.47, 353.16, 353.01, 353.14, 353.18, 352.23, 352.51, 353.22, 352.46, 352.15, 351.51, 351.94, 351.74, 351.74, 351.73, 351.78, 351.49, 351.08, 351.67, 351.61, 351.71, 351.61, 351.28, 351.7, 351.53, 351.68, 351.82, 351.24, 351.07, 352.09, 351.98, 351.86, 352.16, 352.04, 352.28, 352.25, 352.51, 352.27, 352.45, 352.42, 352.54, 352.63, 352.87, 351.53, 352.31, 352.62, 353.02, 353.09, 353.33, 353.99, 353.67, 353.71, 353.45, 353.31, 353.56, 353.69, 353.64, 353.72, 353.27, 353.33, 353.3, 353.36, 353.29, 353.34, 353.73, 353.7, 353.64, 353.52, 353.51, 353.81, 354.01, 354.16, 354.22, 354.09, 354.33, 354.17, 354.23, 354.3, 354.35, 354.59, 354.47, 354.66, 354.6, 354.64, 355.06, 355.42, 355.78, 354.87, 354.87, 355.22, 355.29, 355.2, 354.99, 355.21, 355.81, 355.51, 355.18, 355.08, 355.56, 355.14, 355.22, 355.23, 355.82, 355.4, 355.46, 356.32, 356.12, 356.17, 355.52, 355.72, 356.19, 356.08, 356.36, 355.13, 355.22, 355.24, 356.34, 356.03, 355.88, 355.5, 355.86, 355.58, 355.41, 355.21, 356.32, 356.26, 356.68, 356.44, 356.18, 356.54, 356.18, 356.39, 356.93, 357.18, 356.83, 357.62, 355.99, 356.03, 355.76, 355.78, 356.96, 357.24, 357.29, 357.27, 357.36, 357.98, 358.1, 358.94, 358.24, 357.98, 357.34, 357.9, 358.33, 358.16, 358.44, 358.39, 356.49, 356.71, 358.24, 358.6, 358.53, 358.9, 358.68, 358.76, 358.3, 358.55, 358.94, 359.82, 360.0, 360.09, 359.62, 359.33, 359.09, 358.89, 359.08, 358.77, 358.26, 359.2, 359.33, 359.29, 359.55, 359.4, 359.71, 359.55, 359.77, 359.53, 359.62, 359.71, 359.33, 359.26, 357.89, 357.93, 358.11, 359.75, 358.26, 358.41, 358.59, 360.16, 360.13, 360.15, 360.14, 359.81, 359.93, 360.12, 360.07, 359.92, 359.47, 359.39, 359.21, 359.42, 359.53, 358.33, 358.17, 358.94, 358.98, 358.55, 358.18, 358.17, 358.48, 358.3, 358.17, 358.15, 358.02, 358.33, 358.74, 358.25, 358.34, 355.7, 356.24, 355.63, 355.51, 356.12, 356.28, 354.98, 356.51, 355.86, 356.14, 354.41, 354.5, 355.38, 355.44, 355.71, 355.21, 355.18, 355.35, 355.07, 354.89, 354.8, 355.16, 354.96, 354.78, 354.61, 354.29, 354.5, 353.26, 353.08, 353.15, 353.45, 354.14, 353.05, 352.43, 351.9, 353.59, 353.03, 352.46, 352.26, 351.97, 352.27, 353.65, 353.34, 352.93, 352.76, 353.15, 353.83, 353.75, 353.25, 353.11, 352.26, 352.47, 353.42, 353.78, 353.91, 352.73, 353.0, 352.81, 353.11, 352.78, 352.92, 353.87, 353.61, 354.13, 354.31, 354.33, 354.11, 353.52, 353.3, 353.45, 353.4, 353.83, 353.76, 353.78, 353.71, 353.54, 353.44, 353.85, 354.14, 354.33, 354.31, 354.35, 354.19, 354.89, 354.53, 354.5, 354.74, 354.43, 354.61, 354.66, 354.81, 354.71, 354.64, 354.74, 355.6, 356.01, 354.97, 355.04, 354.99, 355.26, 355.25, 355.0, 354.97, 355.02, 354.96, 356.3, 356.37, 355.29, 355.53, 355.59, 356.17, 356.03, 355.99, 355.65, 356.01, 356.19, 356.38, 357.09, 357.08, 357.18, 356.61, 356.53, 356.48, 356.22, 356.35, 356.5, 356.45, 356.41, 356.61, 356.34, 356.3, 356.5, 357.77, 358.27, 357.49, 357.33, 357.28, 356.12, 356.28, 356.13, 356.01, 356.09, 356.72, 356.87, 356.74, 356.79, 356.99, 357.37, 356.98, 356.49, 356.57, 356.6, 358.08, 358.05, 358.12, 358.34, 358.32, 358.34, 358.58, 358.16, 358.1, 357.96, 356.49, 358.36, 357.74, 357.86, 359.71, 359.81, 358.75, 358.29, 359.09, 359.58, 358.87, 359.03, 358.54, 358.49, 359.72, 359.55, 359.56, 358.3, 358.5, 358.71, 358.86, 359.59, 359.66, 359.62, 359.63, 359.6, 359.71, 359.71, 359.47, 359.49, 358.99, 358.97, 359.36, 359.66, 359.46, 360.47, 360.47, 360.32, 360.74, 359.83, 360.16, 360.92, 360.72, 360.03, 360.31, 360.32, 360.39, 360.36, 359.76, 359.96, 359.98, 359.34, 359.1, 359.4, 359.72, 359.58, 359.7, 359.74, 359.83, 359.83, 359.78, 359.35, 359.57, 358.67, 358.63, 358.33, 358.67, 359.1, 359.18, 359.04, 358.63, 358.49, 357.4, 357.6, 357.3, 357.73, 358.4, 357.88, 357.75, 357.68, 358.35, 358.22, 358.44, 358.44, 358.4, 357.24, 356.1, 356.15, 355.85, 355.73, 354.24, 353.99, 353.55, 353.44, 353.55, 354.16, 353.87, 353.44, 353.82, 354.17, 353.17, 353.44, 354.01, 353.6, 353.65, 353.58, 353.41, 353.68, 353.96, 353.97, 354.21, 354.54, 354.62, 354.45, 354.38, 353.94, 354.32, 353.67, 353.84, 354.46, 354.49, 354.29, 354.24, 354.28, 354.65, 354.71, 354.77, 354.93, 354.88, 354.99, 355.03, 356.16, 356.21, 355.36, 355.4, 355.32, 355.38, 355.94, 356.15, 355.72, 355.61, 355.96, 355.78, 355.75, 356.04, 356.07, 356.96, 356.3, 356.46, 356.81, 356.78, 356.73, 357.4, 356.87, 357.26, 357.3, 357.42, 357.51, 358.17, 358.63, 358.61, 357.62, 357.94, 358.23, 358.03, 358.03, 357.95, 357.96, 358.24, 358.29, 358.16, 358.7, 358.07, 358.11, 358.31, 358.53, 357.95, 357.95, 358.04, 358.18, 358.17, 358.05, 359.44, 359.19, 359.02, 358.59, 357.77, 357.65, 358.51, 358.48, 357.71, 358.76, 358.7, 358.1, 358.02, 358.34, 358.36, 358.54, 358.51, 358.52, 358.98, 359.12, 359.96, 359.74, 358.74, 359.53, 359.86, 359.78, 357.89, 357.84, 358.88, 359.03, 361.07, 360.22, 359.73, 360.34, 361.49, 361.51, 360.29, 360.32, 360.06, 360.7, 360.85, 361.1, 360.53, 360.6, 361.05, 360.61, 360.66, 361.02, 360.74, 360.93, 361.75, 361.19, 362.27, 362.01, 362.15, 361.87, 361.47, 360.33, 360.76, 361.0, 360.91, 361.19, 361.34, 361.43, 361.46, 361.61, 361.67, 361.95, 361.05, 361.08, 361.14, 360.81, 360.27, 360.64, 361.15, 360.85, 360.73, 360.95, 360.96, 360.61, 360.59, 360.68, 360.64, 360.51, 360.36, 360.3, 359.93, 359.97, 360.55, 359.83, 359.75, 359.75, 359.42, 360.63, 360.21, 359.61, 359.53, 358.42, 358.58, 358.51, 357.41, 357.42, 358.04, 357.4, 357.47, 357.52, 357.51, 356.69, 356.73, 355.84, 356.19, 356.07, 356.09, 355.83, 356.35, 356.35, 356.5, 356.73, 356.86, 356.56, 355.34, 355.55, 353.68, 354.77, 356.06, 355.9, 355.82, 355.16, 355.41, 355.32, 355.22, 355.22, 354.91, 355.17, 355.39, 355.47, 355.41, 355.44, 355.53, 355.36, 355.57, 355.57, 355.73, 355.67, 356.0, 355.72, 355.84, 356.12, 354.77, 356.66, 356.57, 356.53, 356.41, 356.5, 356.83, 356.48, 356.31, 356.29, 356.6, 356.52, 356.89, 356.29, 356.94, 356.92, 356.39, 357.41, 357.23, 358.88, 358.77, 358.22, 357.42, 357.61, 357.43, 357.69, 357.52, 357.64, 358.03, 358.19, 358.15, 357.89, 358.04, 358.12, 357.78, 358.56, 358.73, 358.63, 358.15, 358.07, 359.72, 359.28, 359.36, 359.26, 359.0, 359.08, 359.09, 359.79, 359.21, 359.06, 358.87, 358.64, 358.85, 358.62, 358.62, 358.66, 358.54, 359.38, 359.25, 358.89, 359.21, 359.72, 359.54, 359.37, 359.62, 359.84, 359.63, 360.67, 359.34, 359.38, 359.4, 359.81, 359.38, 359.59, 360.09, 360.92, 359.86, 359.85, 359.96, 359.86, 359.96, 360.19, 360.18, 359.89, 359.95, 359.81, 359.83, 359.85, 359.88, 359.93, 359.98, 360.2, 360.78, 360.11, 360.06, 359.92, 361.84, 361.72, 360.4, 360.14, 360.76, 361.96, 361.78, 361.34, 360.96, 360.65, 360.71, 360.92, 360.64, 360.53, 360.51, 360.6, 360.61, 360.96, 361.45, 361.71, 360.77, 361.03, 362.05, 362.36, 361.38, 362.15, 361.8, 362.26, 362.66, 362.06, 361.31, 360.77, 361.23, 361.06, 360.68, 361.22, 361.39, 361.14, 360.54, 360.63, 360.92, 361.73, 361.23, 361.11, 361.32, 362.09, 361.84, 362.0, 361.44, 361.83, 363.35, 363.55, 364.2, 364.1, 363.43, 363.41, 364.07, 362.53, 362.79, 362.89, 362.61, 362.48, 362.42, 363.06, 363.58, 363.55, 363.36, 363.66, 361.9, 363.4, 362.75, 362.98, 363.8, 362.51, 362.37, 363.09, 363.34, 363.32, 363.85, 363.93, 363.92, 364.41, 364.54, 364.68, 364.6, 364.09, 363.95, 363.6, 363.79, 363.69, 363.05, 363.58, 362.96, 364.09, 363.95, 364.04, 363.71, 363.62, 362.6, 363.09, 362.81, 361.32, 361.71, 362.76, 362.72, 363.19, 362.97, 362.8, 362.59, 362.45, 362.32, 361.95, 362.0, 362.27, 362.2, 362.17, 362.07, 362.27, 362.22, 362.16, 361.93, 361.9, 361.88, 361.76, 361.79, 361.15, 361.28, 360.04, 360.62, 360.59, 360.7, 360.61, 360.36, 360.17, 359.11, 359.1, 358.91, 359.11, 359.26, 357.87, 358.33, 358.33, 359.35, 359.26, 359.36, 359.38, 358.38, 358.31, 358.59, 358.36, 358.33, 357.54, 357.84, 358.6, 358.32, 357.78, 357.1, 356.91, 356.94, 357.13, 357.47, 357.54, 357.66, 357.57, 357.48, 357.32, 357.51, 357.21, 357.34, 357.94, 357.6, 357.3, 357.33, 357.3, 357.51, 357.61, 357.91, 357.98, 357.99, 357.87, 357.81, 357.62, 357.77, 357.84, 357.94, 358.6, 358.95, 359.06, 359.27, 359.04, 358.92, 358.92, 359.08, 359.2, 358.65, 359.17, 359.26, 359.14, 359.25, 359.48, 359.42, 359.6, 359.91, 360.02, 360.07, 359.72, 359.79, 359.83, 359.88, 359.9, 360.19, 360.11, 360.24, 360.36, 360.37, 360.29, 360.26, 360.23, 360.29, 360.61, 360.66, 360.34, 360.31, 360.29, 360.45, 360.61, 360.52, 360.7, 360.61, 360.87, 361.51, 361.75, 361.09, 361.72, 361.4, 361.57, 361.47, 361.69, 362.35, 361.54, 362.41, 362.42, 362.04, 361.68, 361.66, 361.92, 361.94, 362.0, 361.92, 361.64, 361.84, 362.05, 362.22, 362.1, 362.09, 362.32, 362.83, 362.2, 361.99, 362.93, 363.13, 362.46, 363.0, 362.75, 362.29, 362.7, 362.7, 363.04, 362.65, 363.43, 363.33, 362.99, 363.33, 363.94, 362.99, 363.04, 363.54, 363.44, 363.85, 363.63, 363.33, 363.59, 364.84, 364.95, 364.32, 364.06, 364.02, 364.13, 364.05, 364.04, 362.52, 363.72, 363.94, 364.03, 364.45, 364.61, 364.88, 364.82, 364.76, 364.65, 365.26, 365.0, 364.86, 364.95, 365.56, 363.7, 363.97, 364.08, 364.2, 365.5, 365.95, 365.34, 365.35, 365.82, 366.06, 365.22, 365.38, 365.5, 365.44, 365.6, 365.56, 364.92, 364.93, 364.51, 364.39, 364.71, 364.71, 364.68, 364.55, 364.55, 364.72, 364.77, 365.05, 364.99, 364.64, 365.49, 365.31, 365.18, 365.4, 365.38, 364.3, 364.36, 364.14, 364.0, 363.94, 364.3, 364.18, 364.54, 364.53, 364.05, 363.78, 363.79, 364.26, 364.08, 362.89, 363.76, 363.76, 363.51, 363.47, 363.51, 362.81, 362.94, 363.19, 362.68, 362.63, 362.52, 361.67, 361.75, 362.7, 362.8, 362.13, 362.12, 361.99, 362.21, 361.76, 361.53, 361.67, 360.92, 360.99, 360.83, 360.81, 360.86, 359.83, 360.19, 360.56, 360.93, 360.64, 360.71, 359.75, 359.65, 360.27, 360.45, 359.69, 360.27, 360.2, 358.64, 358.73, 357.48, 359.28, 359.28, 359.44, 359.41, 360.47, 360.46, 359.92, 360.15, 360.31, 360.67, 360.56, 360.44, 360.43, 360.39, 360.39, 360.8, 360.97, 360.96, 361.03, 361.23, 361.29, 361.68, 361.66, 361.84, 361.82, 361.84, 361.85, 362.87, 362.42, 362.38, 362.33, 362.25, 362.13, 362.04, 361.94, 362.38, 362.77, 363.06, 362.58, 362.67, 362.72, 362.87, 362.51, 362.96, 362.92, 363.41, 363.35, 362.79, 362.42, 363.0, 363.15, 363.01, 362.82, 362.54, 363.12, 363.55, 363.2, 362.9, 363.96, 364.41, 363.34, 362.92, 362.72, 362.83, 363.08, 362.65, 362.64, 363.32, 363.36, 362.32, 362.01, 364.0, 364.26, 364.26, 364.31, 364.32, 364.04, 364.06, 363.99, 363.88, 363.36, 363.17, 363.1, 365.98, 363.61, 363.73, 363.83, 364.15, 364.02, 363.83, 363.83, 363.94, 364.62, 364.37, 364.4, 364.6, 365.3, 365.25, 365.26, 365.12, 365.19, 365.47, 365.35, 365.26, 365.75, 365.64, 365.87, 365.9, 365.59, 365.75, 366.17, 366.28, 366.0, 366.73, 367.07, 366.48, 366.56, 366.96, 366.62, 366.17, 365.68, 365.2, 365.24, 364.96, 365.19, 364.98, 365.14, 364.89, 364.99, 364.85, 364.2, 363.89, 364.11, 364.36, 363.83, 363.64, 363.28, 363.92, 363.92, 363.27, 363.19, 361.26, 361.25, 361.19, 360.98, 362.27, 362.03, 361.77, 361.65, 359.53, 360.09, 360.14, 358.64, 360.1, 360.09, 359.61, 359.01, 358.89, 360.24, 359.99, 360.28, 360.08, 360.27, 360.49, 360.96, 360.85, 360.75, 361.11, 361.14, 360.87, 361.13, 361.09, 360.95, 361.31, 362.06, 361.97, 362.05, 362.07, 361.89, 362.38, 362.15, 361.67, 362.06, 362.26, 362.02, 361.74, 361.89, 362.24, 363.07, 362.97, 362.75, 362.77, 363.34, 363.55, 363.32, 363.65, 363.71, 363.61, 363.81, 363.81, 363.79, 363.72, 363.7, 363.78, 363.75, 363.77, 363.67, 363.9, 364.19, 364.11, 364.12, 364.38, 365.12, 364.84, 364.55, 364.94, 365.47, 364.72, 364.95, 364.61, 364.74, 365.94, 365.79, 364.87, 364.91, 365.56, 366.03, 364.82, 364.8, 365.51, 365.28, 365.07, 365.14, 365.19, 364.76, 365.12, 365.19, 365.22, 365.43, 365.67, 364.84, 364.78, 365.1, 365.05, 364.73, 365.9, 366.03, 366.33, 366.52, 366.07, 365.5, 365.53, 365.4, 365.61, 365.41, 365.43, 365.18, 364.75, 364.97, 365.33, 365.22, 366.93, 366.8, 366.51, 366.31, 367.17, 367.09, 366.1, 366.06, 366.67, 367.59, 367.62, 366.83, 366.9, 366.92, 369.11, 368.97, 367.72, 367.7, 368.33, 368.73, 368.67, 368.68, 368.61, 368.04, 367.99, 368.82, 368.79, 368.51, 368.36, 368.53, 368.04, 367.54, 367.35, 367.28, 368.34, 368.49, 368.64, 368.63, 368.69, 368.95, 368.81, 368.78, 368.89, 368.8, 368.81, 369.35, 369.14, 369.31, 367.94, 368.15, 368.26, 368.25, 368.28, 368.35, 368.55, 369.39, 369.35, 369.43, 369.24, 369.84, 369.56, 369.41, 369.59, 369.82, 369.61, 369.71, 369.14, 369.23, 369.41, 368.93, 369.04, 369.1, 369.02, 368.6, 368.95, 368.43, 368.15, 368.12, 368.68, 368.52, 368.49, 368.3, 368.17, 367.99, 367.77, 368.97, 368.97, 367.62, 367.62, 368.76, 367.11, 367.27, 367.34, 367.82, 367.62, 367.66, 367.46, 367.74, 366.04, 366.82, 366.74, 366.42, 366.61, 365.71, 365.25, 365.96, 365.93, 364.07, 364.13, 364.69, 364.84, 364.33, 364.3, 364.72, 364.43, 363.06, 363.48, 363.81, 362.88, 363.04, 363.19, 363.21, 363.44, 363.44, 363.29, 363.72, 363.78, 363.43, 363.8, 364.14, 364.07, 363.75, 363.96, 363.78, 363.83, 363.82, 364.08, 364.22, 364.27, 364.19, 364.27, 364.44, 364.06, 364.43, 364.54, 364.59, 364.51, 364.54, 364.29, 364.24, 364.56, 364.4, 364.35, 364.5, 364.7, 364.91, 364.61, 364.93, 365.01, 365.07, 365.29, 365.28, 365.11, 365.62, 365.96, 365.45, 365.56, 365.62, 365.49, 365.6, 365.53, 365.73, 365.83, 365.92, 365.65, 365.32, 367.15, 367.12, 366.42, 366.37, 367.37, 367.35, 366.55, 366.51, 366.72, 366.89, 367.1, 367.41, 367.47, 366.53, 366.85, 367.08, 366.87, 367.27, 367.64, 367.29, 366.98, 367.04, 367.03, 367.56, 367.5, 367.53, 367.52, 367.65, 367.52, 368.13, 368.09, 367.6, 367.61, 367.61, 367.58, 367.43, 367.5, 367.34, 367.72, 367.87, 367.82, 368.08, 369.55, 369.58, 369.13, 369.07, 368.99, 369.22, 369.09, 369.18, 370.08, 369.21, 369.04, 368.56, 368.77, 368.81, 369.36, 369.46, 368.58, 368.68, 369.5, 368.34, 368.34, 368.3, 368.12, 367.67, 369.27, 369.28, 369.66, 368.78, 368.52, 368.27, 368.24, 368.53, 368.91, 367.8, 367.78, 367.79, 367.28, 367.85, 368.11, 368.51, 369.24, 368.46, 368.55, 370.59, 368.39, 369.05, 368.68, 368.75, 371.78, 369.99, 369.86, 370.11, 370.1, 370.27, 370.65, 370.72, 370.7, 370.96, 371.9, 372.13, 371.55, 371.34, 371.23, 371.11, 370.82, 370.88, 371.23, 370.77, 370.58, 370.71, 368.86, 369.04, 371.02, 370.96, 369.95, 369.77, 371.46, 371.51, 371.3, 371.36, 371.22, 371.01, 370.9, 371.1, 370.35, 370.46, 370.59, 371.41, 370.26, 369.94, 370.21, 370.08, 370.05, 370.17, 370.76, 370.31, 371.05, 370.14, 370.24, 370.69, 370.23, 371.13, 370.98, 369.76, 370.04, 370.25, 370.27, 370.09, 369.83, 369.94, 369.93, 369.92, 369.78, 368.93, 368.82, 369.15, 370.41, 370.03, 370.38, 370.18, 370.56, 370.75, 370.98, 370.81, 368.6, 368.85, 368.91, 368.04, 368.14, 368.39, 368.76, 367.36, 367.36, 366.85, 367.55, 367.37, 367.54, 368.12, 367.47, 367.41, 366.67, 366.8, 366.68, 366.28, 366.51, 366.62, 366.45, 366.58, 366.15, 365.82, 366.84, 366.88, 365.52, 366.15, 366.3, 363.39, 363.2, 363.26, 363.5, 364.52, 364.63, 364.47, 364.39, 365.44, 365.42, 365.2, 365.13, 365.05, 365.12, 364.61, 364.47, 364.47, 364.37, 364.5, 364.35, 364.27, 364.04, 364.2, 364.16, 364.54, 364.49, 365.04, 365.01, 365.07, 364.72, 364.81, 365.11, 365.12, 364.89, 364.45, 364.45, 365.33, 364.7, 364.52, 366.09, 365.8, 365.95, 365.68, 365.35, 365.2, 365.38, 365.69, 365.62, 366.12, 366.16, 365.69, 366.01, 366.18, 366.76, 366.4, 366.09, 366.72, 366.99, 366.31, 366.77, 366.71, 366.49, 366.47, 367.4, 367.0, 366.87, 367.03, 367.15, 367.16, 368.08, 367.33, 367.37, 367.42, 368.4, 368.06, 368.02, 368.3, 367.89, 367.71, 367.76, 367.63, 367.74, 367.86, 368.24, 367.71, 368.33, 368.23, 368.19, 367.8, 367.79, 367.96, 368.56, 368.48, 368.67, 368.8, 368.75, 368.55, 368.53, 368.56, 368.59, 368.36, 368.23, 368.58, 368.73, 368.57, 368.45, 368.91, 368.74, 368.82, 369.0, 369.93, 369.81, 369.76, 369.84, 369.47, 369.98, 369.27, 369.11, 369.29, 369.27, 369.13, 369.22, 369.34, 369.42, 369.67, 369.69, 370.4, 368.94, 369.0, 368.55, 368.74, 369.3, 369.92, 369.79, 369.91, 369.36, 369.94, 369.41, 370.03, 370.01, 369.42, 369.81, 370.33, 371.3, 370.41, 370.63, 370.67, 370.16, 370.57, 371.12, 370.99, 370.57, 370.12, 371.41, 372.04, 371.22, 373.02, 372.57, 372.12, 371.99, 372.03, 372.94, 373.01, 371.61, 371.51, 371.71, 371.66, 371.71, 371.71, 370.64, 370.57, 371.14, 370.64, 371.19, 371.66, 371.73, 371.91, 371.96, 372.14, 371.38, 371.37, 372.24, 372.19, 372.22, 371.69, 371.72, 371.46, 371.41, 371.78, 372.16, 372.17, 372.12, 372.21, 371.82, 371.92, 371.83, 371.78, 371.55, 371.56, 371.84, 371.7, 371.59, 371.62, 371.57, 371.88, 371.73, 372.06, 371.96, 371.46, 371.22, 371.01, 371.28, 371.05, 371.04, 370.2, 370.02, 370.27, 370.12, 370.4, 370.34, 370.37, 370.22, 370.34, 370.14, 369.88, 370.03, 370.11, 370.04, 370.02, 369.63, 369.04, 369.36, 369.17, 368.69, 368.98, 368.66, 369.13, 369.07, 368.55, 369.2, 369.17, 368.61, 368.8, 367.7, 367.65, 366.97, 367.16, 367.26, 367.05, 367.23, 366.96, 367.02, 367.35, 366.04, 366.0, 366.43, 366.22, 366.09, 366.18, 366.56, 366.66, 365.93, 365.83, 366.2, 366.5, 366.51, 366.63, 366.87, 366.78, 366.42, 366.52, 366.57, 366.62, 366.45, 366.97, 366.53, 366.45, 367.13, 367.39, 367.52, 367.54, 367.65, 367.6, 367.76, 367.64, 367.74, 367.79, 367.99, 367.71, 367.89, 367.84, 368.68, 369.01, 368.36, 368.42, 369.49, 369.25, 368.14, 368.15, 368.27, 368.46, 368.4, 368.63, 368.94, 369.96, 369.41, 369.6, 369.55, 369.36, 369.29, 369.34, 369.34, 369.62, 369.55, 369.63, 369.79, 369.08, 369.67, 369.54, 369.81, 369.71, 369.7, 369.73, 369.85, 370.24, 369.84, 369.94, 369.96, 369.82, 369.8, 369.86, 369.8, 371.18, 371.22, 370.11, 370.58, 369.62, 369.86, 369.69, 369.7, 369.61, 369.85, 370.86, 371.37, 371.49, 371.08, 371.43, 371.29, 371.04, 370.87, 370.87, 372.35, 370.66, 370.51, 371.41, 371.46, 371.28, 371.76, 371.76, 371.85, 371.36, 371.48, 371.24, 371.55, 371.54, 371.42, 370.9, 370.59, 372.24, 371.72, 371.41, 372.36, 372.16, 371.85, 372.03, 372.35, 372.17, 371.51, 371.89, 371.96, 372.44, 372.57, 374.77, 375.02, 372.07, 372.28, 372.42, 372.89, 370.97, 373.01, 373.93, 374.19, 374.24, 374.34, 374.02, 373.76, 374.13, 374.32, 373.74, 374.26, 374.31, 372.91, 373.11, 373.2, 372.74, 372.66, 372.79, 373.26, 373.05, 372.73, 372.8, 372.93, 372.89, 373.19, 372.43, 372.65, 372.64, 372.87, 371.53, 371.49, 371.56, 369.45, 370.45, 370.63, 369.26, 370.19, 370.25, 370.11, 369.7, 369.9, 369.82, 369.35, 369.33, 368.92, 369.35, 367.36, 368.52, 368.46, 367.83, 368.0, 368.89, 368.72, 367.51, 367.57, 367.56, 367.53, 367.59, 368.28, 368.54, 367.26, 367.02, 367.83, 367.47, 367.38, 368.25, 367.57, 367.67, 368.57, 368.49, 368.23, 367.53, 367.89, 367.95, 367.95, 368.72, 368.61, 368.74, 369.22, 368.71, 368.47, 368.73, 368.95, 369.06, 370.35, 370.18, 369.7, 369.84, 369.57, 370.58, 370.47, 370.12, 370.52, 370.61, 370.87, 370.67, 372.07, 371.78, 371.52, 370.95, 371.05, 371.08, 370.93, 371.22, 371.5, 371.48, 371.46, 371.34, 371.29, 371.17, 371.08, 372.24, 372.53, 372.34, 373.34, 373.55, 373.11, 373.21, 372.99, 372.63, 372.04, 371.98, 372.19, 373.25, 372.83, 372.84, 372.62, 372.53, 372.59, 372.15, 374.28, 374.0, 372.56, 373.74, 373.22, 372.88, 373.87, 374.01, 373.51, 373.53, 372.87, 373.06, 373.2, 373.0, 373.1, 373.48, 373.51, 374.36, 375.17, 374.59, 373.99, 374.04, 373.96, 375.63, 375.5, 375.19, 375.03, 374.34, 375.19, 375.08, 374.82, 374.7, 375.06, 375.73, 375.85, 375.88, 376.15, 376.03, 375.4, 375.91, 374.37, 375.12, 374.38, 374.85, 375.42, 375.42, 375.69, 375.93, 375.95, 375.87, 375.73, 375.76, 376.05, 375.74, 375.79, 375.97, 375.63, 375.41, 375.4, 375.29, 376.25, 376.26, 375.72, 375.52, 375.4, 375.03, 375.21, 375.22, 374.56, 375.07, 374.93, 374.9, 376.91, 376.85, 374.4, 374.45, 374.37, 374.69, 374.68, 374.54, 374.7, 374.58, 374.28, 374.2, 374.39, 373.51, 373.61, 374.16, 373.36, 373.53, 373.03, 373.35, 373.4, 373.41, 373.16, 372.32, 372.06, 371.68, 371.1, 371.07, 371.56, 371.49, 371.42, 371.52, 371.3, 371.28, 370.01, 370.01, 371.34, 371.44, 369.49, 369.33, 371.39, 369.62, 369.93, 370.81, 370.85, 370.61, 370.24, 369.74, 369.0, 369.32, 369.21, 369.34, 369.52, 369.48, 370.05, 370.0, 370.15, 371.34, 371.01, 370.93, 371.95, 371.14, 370.68, 370.33, 370.34, 371.23, 370.93, 371.14, 371.18, 372.51, 372.47, 371.52, 371.34, 371.4, 371.31, 371.45, 371.71, 371.64, 371.56, 371.54, 372.55, 372.29, 372.47, 372.34, 373.02, 373.15, 372.85, 372.36, 372.26, 372.39, 373.55, 373.68, 372.84, 373.78, 373.88, 373.22, 373.16, 373.07, 373.31, 374.26, 374.68, 373.84, 373.82, 373.5, 374.73, 374.73, 374.81, 374.85, 374.91, 373.98, 373.81, 374.17, 374.4, 373.61, 374.02, 374.37, 374.63, 374.77, 374.46, 374.4, 374.3, 374.83, 374.87, 374.16, 375.51, 375.79, 374.98, 374.81, 374.4, 374.63, 375.29, 374.94, 374.81, 374.99, 374.85, 375.49, 375.35, 375.08, 374.99, 375.07, 374.56, 374.45, 375.02, 375.35, 374.78, 374.56, 374.6, 374.66, 374.58, 375.5, 376.21, 376.14, 376.16, 375.68, 375.88, 376.16, 376.32, 376.38, 376.81, 376.7, 375.31, 375.35, 375.16, 375.83, 376.92, 375.94, 375.72, 375.93, 374.72, 376.02, 375.59, 375.35, 375.79, 375.35, 375.67, 375.8, 375.87, 376.23, 376.14, 376.23, 376.27, 376.33, 376.45, 376.71, 377.35, 377.43, 377.51, 376.85, 376.44, 375.92, 377.12, 376.86, 377.28, 376.92, 376.42, 376.4, 376.85, 377.07, 377.33, 376.76, 376.91, 377.5, 377.41, 377.9, 377.73, 377.64, 377.83, 378.27, 378.05, 378.25, 378.11, 378.95, 379.14, 378.14, 377.81, 377.76, 377.34, 377.32, 377.26, 377.45, 377.61, 377.67, 377.46, 377.29, 377.18, 377.35, 377.21, 379.1, 379.48, 379.79, 379.96, 378.7, 378.38, 378.43, 378.51, 378.51, 378.64, 377.27, 377.54, 378.36, 378.49, 377.94, 377.86, 377.87, 377.23, 378.81, 377.64, 377.65, 377.74, 377.97, 375.87, 376.41, 376.44, 376.08, 375.71, 375.92, 376.4, 376.99, 377.51, 378.18, 377.01, 376.73, 376.94, 377.14, 375.1, 376.67, 376.78, 376.63, 376.53, 375.53, 375.55, 375.88, 376.0, 376.21, 375.74, 373.58, 375.68, 375.1, 374.68, 374.93, 375.2, 375.13, 374.68, 374.11, 373.65, 375.12, 375.09, 375.1, 374.61, 375.36, 373.68, 373.93, 372.12, 373.63, 374.06, 374.55, 374.78, 374.71, 374.79, 374.07, 373.24, 373.4, 374.62, 372.16, 372.02, 373.07, 372.91, 372.5, 372.9, 372.94, 373.28, 373.08, 372.91, 372.87, 372.98, 372.86, 373.0, 373.65, 372.92, 373.16, 372.97, 373.35, 373.52, 373.36, 373.05, 373.97, 374.59, 374.41, 374.48, 374.08, 375.55, 375.06, 375.11, 375.57, 375.37, 375.21, 375.11, 374.78, 374.84, 374.32, 374.5, 374.64, 374.61, 374.57, 374.3, 376.82, 376.08, 376.25, 376.86, 376.7, 376.26, 375.83, 376.13, 376.09, 375.75, 376.19, 376.41, 375.98, 376.02, 376.83, 376.44, 378.1, 379.61, 379.02, 378.69, 378.57, 378.1, 377.92, 378.17, 380.76, 380.62, 380.13, 380.03, 380.31, 380.99, 381.09, 380.53, 379.54, 379.28, 381.25, 381.21, 381.18, 380.1, 380.22, 380.66, 381.12, 380.3, 380.73, 380.27, 379.34, 379.44, 380.11, 380.53, 380.92, 380.85, 380.44, 381.01, 381.02, 379.13, 378.95, 379.93, 379.45, 379.87, 379.74, 380.08, 380.24, 379.81, 378.78, 379.39, 379.16, 378.99, 378.97, 378.97, 378.91, 378.32, 378.67, 378.66, 376.87, 378.1, 378.01, 377.93, 376.26, 376.59, 377.75, 376.58, 376.74, 376.65, 376.66, 375.92, 375.85, 375.91, 375.81, 375.75, 376.01, 376.04, 374.74, 375.87, 375.69, 375.51, 373.91, 373.39, 373.79, 373.67, 374.04, 374.04, 373.89, 374.28, 373.91, 374.33, 374.35, 373.58, 373.73, 373.62, 373.39, 373.79, 373.77, 373.83, 373.94, 374.01, 374.1, 374.13, 373.79, 374.41, 374.71, 374.52, 374.85, 374.56, 374.41, 374.56, 374.76, 374.84, 375.08, 375.44, 375.16, 374.92, 374.86, 374.66, 375.19, 374.87, 374.99, 374.94, 375.76, 375.68, 375.63, 376.46, 378.01, 376.72, 376.5, 377.27, 375.65, 376.27, 376.52, 376.26, 376.39, 376.4, 376.1, 376.01, 375.54, 375.3, 375.62, 377.28, 377.09, 377.21, 377.09, 377.18, 377.61, 377.42, 376.97, 377.47, 378.01, 376.93, 376.97, 377.1, 377.06, 377.26, 377.13, 377.25, 377.05, 377.65, 377.6, 377.34, 377.43, 377.39, 377.07, 377.52, 377.56, 377.64, 378.01, 377.67, 377.8, 378.01, 378.23, 378.28, 377.89, 378.13, 378.05, 378.06, 378.12, 378.52, 378.63, 377.88, 377.73, 378.7, 378.26, 378.19, 378.51, 378.54, 378.98, 378.54, 379.01, 379.05, 379.05, 379.43, 379.43, 378.57, 378.62, 378.8, 378.86, 379.01, 378.64, 379.1, 379.14, 380.01, 379.8, 380.28, 379.94, 379.19, 379.9, 380.97, 380.55, 379.56, 379.64, 379.59, 379.98, 381.25, 380.17, 380.79, 380.63, 380.98, 380.44, 379.62, 378.86, 379.8, 379.49, 380.13, 380.81, 381.49, 381.81, 382.05, 379.95, 380.26, 381.14, 380.77, 380.34, 379.76, 379.96, 378.97, 379.08, 379.22, 382.81, 381.8, 382.37, 382.46, 382.3, 381.85, 381.61, 382.0, 381.69, 382.22, 382.59, 382.32, 381.9, 381.81, 382.7, 381.98, 381.27, 381.4, 381.45, 381.13, 381.03, 381.17, 381.78, 382.11, 382.44, 382.65, 381.3, 381.76, 381.96, 382.88, 382.74, 382.7, 381.46, 381.38, 381.6, 381.39, 381.39, 381.86, 381.19, 381.54, 382.1, 382.61, 382.3, 382.43, 382.9, 383.07, 382.09, 382.11, 382.27, 382.55, 382.41, 382.78, 382.84, 382.46, 382.57, 382.69, 382.01, 382.39, 382.21, 382.12, 382.3, 382.29, 382.23, 382.51, 382.4, 382.17, 382.12, 382.21, 382.33, 382.09, 382.07, 382.38, 381.98, 381.97, 381.81, 381.58, 381.69, 381.63, 381.5, 381.31, 381.3, 381.16, 381.23, 381.27, 381.26, 381.08, 380.91, 380.95, 381.12, 381.12, 380.98, 381.01, 380.96, 379.78, 380.87, 381.38, 381.53, 380.1, 379.9, 380.71, 380.74, 380.58, 380.63, 380.53, 380.67, 380.64, 380.24, 380.29, 380.29, 379.6, 379.81, 379.87, 379.94, 379.99, 379.47, 379.91, 379.89, 379.38, 379.81, 378.34, 378.43, 378.48, 378.97, 379.13, 379.0, 379.1, 378.94, 378.82, 378.64, 378.34, 378.42, 378.22, 377.76, 377.54, 377.64, 377.48, 377.69, 377.95, 378.2, 377.9, 377.6, 377.58, 377.44, 377.07, 376.36, 376.27, 376.2, 376.22, 375.73, 376.21, 376.02, 376.04, 376.42, 376.51, 376.08, 376.13, 375.53, 374.88, 375.42, 375.96, 376.29, 376.0, 376.18, 376.68, 376.55, 376.31, 376.1, 376.16, 376.38, 376.13, 376.48, 376.42, 376.36, 376.36, 376.41, 376.56, 376.42, 376.33, 376.84, 377.3, 377.23, 377.24, 377.3, 377.02, 377.25, 377.27, 377.3, 377.8, 377.84, 377.81, 378.05, 378.02, 378.06, 378.02, 377.84, 377.99, 377.95, 378.09, 378.28, 378.62, 378.8, 378.83, 378.71, 378.5, 378.79, 378.45, 378.59, 378.47, 379.16, 379.05, 379.12, 378.97, 379.14, 379.06, 379.98, 379.11, 379.47, 380.56, 380.12, 379.65, 379.76, 379.81, 379.75, 380.57, 380.42, 379.88, 379.85, 379.87, 380.04, 379.95, 379.92, 380.4, 380.11, 380.06, 380.31, 380.48, 380.54, 380.33, 380.57, 380.59, 380.01, 380.27, 380.77, 381.34, 381.59, 381.16, 381.11, 381.68, 381.1, 381.24, 381.21, 381.09, 381.72, 381.61, 381.47, 381.29, 381.34, 381.38, 381.53, 381.61, 381.64, 381.65, 381.61, 381.18, 381.52, 381.58, 381.72, 381.68, 381.33, 381.06, 381.21, 381.39, 382.03, 382.66, 382.57, 382.13, 382.03, 381.38, 381.78, 381.9, 382.32, 381.76, 381.41, 381.42, 382.65, 382.61, 382.57, 382.95, 382.9, 382.44, 382.65, 382.53, 382.79, 382.43, 382.75, 382.05, 382.49, 382.69, 382.66, 382.71, 383.04, 382.56, 382.81, 382.71, 382.71, 382.21, 382.34, 382.59, 382.23, 382.2, 382.49, 382.3, 382.84, 382.77, 383.96, 383.98, 383.65, 383.57, 384.76, 385.41, 385.53, 384.49, 384.93, 384.66, 384.35, 384.4, 384.78, 384.89, 384.72, 384.68, 384.81, 384.93, 384.51, 384.49, 384.62, 384.64, 384.79, 384.75, 384.3, 384.61, 384.46, 384.07, 385.35, 385.09, 384.63, 384.44, 384.89, 385.03, 385.16, 384.92, 385.11, 384.41, 384.68, 384.85, 384.96, 384.93, 384.96, 385.36, 385.32, 385.22, 385.12, 385.11, 385.33, 385.7, 385.06, 384.13, 383.5, 383.37, 384.85, 384.72, 384.97, 384.52, 384.5, 384.53, 384.24, 382.98, 384.08, 384.53, 384.37, 384.17, 384.38, 384.17, 384.22, 384.1, 384.3, 384.47, 384.24, 383.29, 384.21, 384.12, 383.67, 383.57, 383.41, 383.46, 383.35, 383.03, 384.13, 382.92, 383.06, 382.85, 382.85, 381.91, 381.54, 381.62, 381.73, 382.14, 381.62, 381.92, 381.93, 381.57, 381.75, 381.14, 381.02, 381.46, 381.43, 381.58, 381.33, 381.2, 380.64, 380.93, 381.12, 380.98, 380.83, 380.98, 380.61, 379.79, 379.92, 380.3, 380.5, 380.31, 379.92, 379.89, 380.09, 379.82, 379.78, 379.78, 379.55, 379.49, 377.57, 378.22, 378.8, 378.8, 379.01, 379.09, 378.93, 378.87, 378.72, 378.69, 378.79, 378.74, 378.57, 378.42, 378.2, 378.4, 378.61, 378.49, 378.54, 378.73, 379.16, 379.28, 379.18, 378.38, 378.52, 378.99, 378.95, 378.73, 378.66, 378.68, 379.18, 379.55, 379.42, 378.92, 378.98, 379.14, 379.06, 379.28, 379.29, 379.18, 379.19, 379.54, 379.91, 379.74, 379.72, 379.48, 379.59, 379.58, 379.67, 379.65, 379.61, 379.66, 379.72, 379.68, 379.67, 379.8, 379.82, 379.85, 379.91, 379.7, 380.03, 379.96, 380.05, 379.92, 379.85, 379.75, 380.07, 380.89, 381.64, 381.18, 382.04, 381.56, 381.52, 381.88, 381.43, 380.81, 380.81, 381.77, 382.22, 381.82, 383.21, 382.07, 382.32, 382.06, 381.86, 381.81, 381.81, 381.59, 381.69, 381.63, 381.57, 381.53, 381.88, 381.79, 382.01, 381.84, 381.62, 381.78, 383.72, 383.47, 383.21, 382.51, 382.6, 382.56, 382.65, 382.46, 381.78, 381.67, 381.81, 382.74, 383.43, 383.85, 382.99, 382.6, 382.72, 382.81, 382.74, 382.98, 384.35, 384.06, 383.85, 384.21, 384.16, 384.18, 384.49, 384.32, 383.86, 384.17, 383.82, 383.39, 383.2, 383.34, 383.82, 384.55, 384.16, 383.89, 383.71, 383.01, 382.59, 382.63, 383.22, 383.28, 383.51, 384.52, 384.19, 383.89, 383.84, 383.85, 384.43, 384.49, 384.22, 384.68, 385.03, 384.87, 384.19, 385.06, 385.5, 386.39, 386.72, 385.55, 385.95, 386.15, 385.28, 385.34, 385.16, 384.67, 386.0, 386.92, 384.91, 385.15, 385.72, 387.52, 387.37, 387.47, 387.26, 386.9, 386.8, 386.96, 386.61, 386.77, 386.37, 386.37, 386.31, 386.45, 386.67, 386.35, 386.2, 386.41, 385.43, 385.32, 385.43, 385.58, 386.02, 387.15, 386.61, 386.67, 386.94, 386.55, 385.62, 386.21, 386.34, 386.08, 385.03, 386.32, 386.83, 387.07, 386.75, 387.14, 386.85, 386.94, 386.75, 386.49, 386.4, 386.38, 386.47, 386.73, 386.6, 386.91, 386.82, 386.41, 386.42, 386.77, 385.76, 385.69, 385.56, 386.47, 386.31, 386.09, 386.29, 386.22, 386.19, 385.22, 384.69, 384.91, 384.62, 385.35, 384.75, 384.96, 385.01, 385.15, 384.77, 384.84, 384.77, 384.67, 385.62, 384.88, 384.65, 384.71, 384.39, 384.38, 384.41, 384.26, 383.82, 383.88, 383.78, 383.35, 383.46, 383.84, 383.7, 383.03, 383.12, 383.24, 383.08, 382.12, 380.83, 383.14, 381.74, 381.56, 381.77, 381.25, 382.22, 381.64, 381.49, 381.08, 382.0, 382.14, 381.67, 381.64, 381.11, 381.49, 381.82, 381.64, 381.68, 381.4, 380.86, 380.93, 380.72, 380.67, 380.05, 380.34, 380.58, 380.55, 380.51, 380.77, 381.1, 380.84, 380.78, 381.03, 381.1, 380.76, 380.88, 380.88, 380.67, 380.26, 380.71, 381.01, 381.3, 380.73, 380.71, 380.6, 380.61, 380.6, 380.46, 380.96, 380.66, 380.7, 380.47, 380.58, 380.56, 380.88, 380.71, 381.15, 381.05, 381.01, 380.97, 381.39, 381.13, 381.14, 381.13, 381.14, 381.33, 381.61, 381.54, 381.57, 381.74, 381.54, 381.76, 381.75, 381.81, 381.97, 382.04, 381.92, 381.71, 381.89, 381.55, 382.36, 382.47, 382.45, 382.82, 382.51, 382.61, 383.27, 383.34, 382.94, 382.69, 382.64, 382.66, 382.73, 382.65, 382.76, 382.94, 382.97, 382.93, 383.22, 383.27, 383.42, 383.86, 383.48, 383.38, 383.65, 383.86, 384.33, 383.89, 383.98, 384.05, 383.82, 383.53, 383.78, 384.03, 383.68, 383.69, 383.76, 383.92, 385.0, 384.8, 384.44, 384.14, 384.32, 384.06, 384.21, 384.19, 384.05, 384.1, 385.18, 385.42, 385.45, 385.51, 385.64, 385.96, 386.56, 386.36, 386.16, 386.25, 386.43, 386.45, 386.11, 386.1, 385.97, 385.8, 385.33, 385.38, 385.74, 386.1, 386.18, 385.47, 385.65, 385.45, 385.54, 385.69, 385.68, 385.71, 385.24, 385.21, 385.2, 385.3, 385.12, 384.59, 384.43, 384.52, 384.99, 384.78, 385.78, 386.11, 386.22, 386.1, 385.84, 385.65, 385.56, 385.5, 385.5, 385.78, 385.33, 385.52, 385.38, 386.04, 385.69, 385.63, 385.59, 385.46, 385.64, 385.88, 387.38, 387.42, 387.37, 385.77, 385.99, 386.11, 387.32, 387.47, 388.14, 388.2, 387.42, 387.99, 388.08, 385.6, 385.55, 385.7, 385.74, 386.2, 386.16, 386.12, 386.69, 386.16, 386.17, 386.58, 386.28, 386.24, 386.69, 387.92, 386.72, 386.68, 387.51, 386.58, 386.86, 389.21, 389.21, 389.1, 388.23, 388.91, 388.67, 388.76, 388.59, 388.73, 389.14, 388.92, 388.6, 388.66, 388.18, 388.39, 388.42, 388.37, 388.33, 388.36, 388.56, 388.7, 388.59, 388.48, 388.18, 388.27, 388.18, 388.6, 388.59, 386.99, 386.93, 386.79, 387.9, 388.6, 388.07, 387.86, 388.16, 387.98, 388.06, 387.32, 387.4, 387.06, 387.24, 387.08, 386.03, 386.01, 386.0, 386.25, 385.9, 385.5, 384.78, 384.46, 384.94, 384.96, 384.87, 385.23, 384.88, 384.94, 385.17, 385.38, 382.89, 383.15, 382.81, 383.54, 384.7, 384.66, 384.17, 383.91, 383.68, 383.04, 382.84, 383.14, 383.2, 383.39, 383.08, 383.12, 383.37, 383.61, 383.59, 383.8, 382.07, 382.45, 383.33, 383.33, 383.42, 383.31, 383.28, 383.14, 383.01, 382.3, 383.18, 383.02, 382.93, 383.08, 383.27, 383.13, 382.84, 382.76, 382.77, 383.18, 382.27, 382.31, 382.69, 382.84, 382.35, 382.95, 382.99, 382.75, 382.57, 382.54, 382.67, 382.57, 382.74, 382.69, 382.82, 382.83, 382.97, 383.07, 383.07, 383.06, 382.96, 382.68, 382.58, 383.06, 383.03, 382.99, 383.09, 383.41, 383.24, 383.77, 383.69, 383.73, 383.67, 383.52, 383.42, 383.56, 383.67, 383.78, 383.51, 383.56, 383.76, 384.06, 384.04, 383.94, 384.21, 384.06, 383.96, 384.17, 384.47, 384.27, 384.32, 384.33, 384.18, 384.0, 383.95, 384.32, 384.41, 384.35, 384.42, 385.56, 384.81, 384.81, 384.75, 384.95, 384.36, 384.89, 384.65, 384.59, 384.81, 384.88, 384.92, 385.0, 385.01, 384.96, 385.07, 385.07, 385.05, 385.06, 385.18, 385.44, 385.75, 385.83, 385.9, 385.79, 385.63, 385.5, 385.98, 386.06, 385.85, 385.61, 385.71, 386.05, 386.63, 386.24, 386.11, 385.89, 386.52, 387.57, 387.18, 386.4, 386.66, 386.78, 386.61, 386.68, 386.56, 386.6, 387.34, 387.11, 386.76, 386.71, 386.76, 387.23, 387.23, 387.62, 387.1, 387.12, 387.06, 387.2, 386.9, 386.19, 386.33, 386.47, 386.57, 386.43, 386.52, 386.74, 387.38, 387.45, 388.25, 387.68, 387.03, 387.02, 386.9, 387.21, 387.31, 387.68, 387.77, 387.74, 387.46, 388.52, 389.12, 389.16, 388.75, 388.67, 388.3, 388.25, 388.08, 388.0, 388.29, 388.23, 388.55, 388.88, 388.52, 389.66, 389.59, 389.02, 388.94, 389.14, 388.91, 389.19, 389.32, 389.68, 389.43, 389.52, 389.55, 389.78, 389.58, 389.69, 389.87, 390.07, 389.53, 389.47, 388.68, 388.24, 388.35, 387.94, 388.22, 388.82, 390.27, 389.87, 390.12, 390.97, 390.34, 390.29, 390.48, 390.65, 390.58, 390.07, 389.74, 389.9, 390.15, 389.9, 390.12, 388.95, 389.68, 390.0, 390.1, 390.0, 390.15, 390.59, 390.26, 389.88, 390.17, 390.03, 390.25, 390.78, 390.48, 390.68, 390.55, 390.68, 390.75, 390.98, 391.09, 389.72, 389.5, 390.54, 388.95, 389.02, 388.9, 388.7, 390.52, 390.52, 390.47, 390.48, 390.55, 390.57, 389.98, 390.16, 390.11, 390.0, 390.01, 390.18, 390.28, 389.2, 388.97, 388.73, 388.73, 388.75, 389.05, 388.84, 389.0, 388.14, 388.09, 388.87, 389.03, 389.05, 389.05, 387.95, 388.2, 388.11, 388.34, 388.32, 388.4, 388.58, 388.81, 388.85, 388.13, 388.24, 387.67, 388.01, 387.73, 387.42, 387.72, 387.41, 387.31, 387.18, 387.05, 387.19, 386.98, 387.2, 387.28, 387.11, 387.18, 385.67, 385.42, 385.24, 386.03, 385.09, 384.78, 384.95, 386.27, 385.82, 386.18, 386.25, 385.76, 385.95, 386.02, 385.47, 385.39, 385.37, 384.79, 385.51, 385.05, 384.24, 384.1, 384.31, 384.59, 384.5, 384.6, 385.07, 383.88, 384.92, 384.83, 384.67, 384.47, 384.32, 384.41, 384.27, 383.09, 382.94, 383.46, 383.77, 383.79, 383.76, 383.95, 384.53, 384.29, 384.59, 384.52, 384.39, 384.36, 384.41, 384.5, 384.76, 384.71, 384.71, 384.57, 384.78, 384.83, 384.73, 384.95, 384.88, 385.13, 385.05, 385.18, 385.25, 385.25, 385.14, 385.21, 385.38, 385.82, 385.66, 385.44, 385.68, 385.47, 385.74, 385.79, 385.74, 385.95, 386.1, 386.14, 386.81, 386.31, 386.3, 386.24, 386.2, 386.39, 386.42, 386.16, 386.33, 386.91, 386.89, 387.04, 387.0, 386.77, 386.65, 387.09, 386.96, 386.68, 386.95, 387.07, 386.98, 386.76, 386.61, 386.93, 386.79, 386.88, 386.87, 386.9, 386.89, 389.59, 388.4, 388.18, 388.28, 389.11, 388.64, 387.55, 387.75, 387.82, 387.64, 387.68, 387.66, 390.61, 388.12, 388.21, 388.19, 388.02, 387.97, 388.13, 388.77, 388.24, 388.05, 387.99, 388.13, 389.34, 388.77, 388.68, 388.68, 388.55, 388.65, 388.55, 388.13, 388.03, 388.01, 388.57, 389.34, 389.5, 389.22, 389.07, 388.53, 388.99, 388.59, 389.17, 389.72, 389.76, 391.54, 391.45, 389.87, 390.14, 389.85, 390.14, 389.92, 389.28, 389.24, 390.67, 391.56, 390.89, 390.68, 391.56, 389.68, 388.94, 388.61, 389.01, 389.49, 390.86, 391.34, 391.09, 390.82, 391.7, 391.53, 391.44, 391.13, 391.32, 391.26, 391.21, 391.84, 392.43, 391.8, 392.07, 389.67, 389.82, 389.97, 389.79, 390.77, 390.69, 391.2, 391.29, 391.34, 391.28, 391.12, 391.51, 391.14, 391.47, 391.72, 392.54, 392.67, 392.46, 393.65, 393.87, 393.74, 393.21, 393.15, 392.94, 393.23, 393.56, 393.56, 393.16, 393.52, 393.37, 393.14, 391.86, 393.25, 393.06, 393.02, 392.91, 393.14, 392.54, 392.39, 393.8, 393.49, 393.63, 393.5, 393.32, 393.45, 393.61, 393.7, 393.83, 393.52, 393.4, 392.52, 393.61, 394.09, 393.87, 393.24, 393.24, 392.82, 392.93, 393.2, 393.14, 393.1, 393.04, 393.14, 392.74, 392.42, 392.07, 391.46, 391.71, 392.63, 391.14, 391.79, 391.41, 391.34, 390.69, 390.71, 391.63, 391.32, 391.31, 390.86, 390.68, 389.5, 389.66, 391.04, 390.7, 390.19, 389.58, 389.61, 390.58, 390.02, 390.54, 390.04, 390.02, 390.03, 390.08, 390.08, 389.82, 389.94, 388.92, 388.21, 388.97, 389.32, 389.02, 388.71, 389.17, 389.24, 388.88, 388.54, 388.79, 388.94, 388.87, 388.86, 388.54, 388.58, 388.5, 388.49, 388.39, 387.78, 387.46, 387.71, 387.68, 387.52, 387.39, 387.24, 387.36, 387.57, 387.68, 387.89, 387.7, 385.43, 385.23, 385.98, 387.19, 387.15, 387.01, 386.99, 386.84, 387.11, 387.3, 387.33, 386.8, 386.66, 386.71, 386.97, 386.69, 386.89, 386.87, 386.84, 387.0, 386.98, 386.9, 386.81, 386.68, 386.78, 386.59, 386.84, 386.88, 386.75, 387.32, 387.13, 387.07, 386.81, 387.02, 386.92, 386.67, 386.87, 387.03, 386.94, 386.89, 387.18, 387.3, 387.64, 387.47, 388.01, 388.01, 387.93, 387.95, 387.37, 387.64, 387.92, 387.83, 387.94, 387.93, 388.01, 387.96, 388.16, 387.69, 387.8, 387.81, 388.12, 388.29, 388.0, 388.04, 388.26, 388.48, 388.65, 388.31, 388.38, 388.77, 388.93, 389.66, 389.64, 389.46, 389.16, 389.31, 389.69, 389.87, 389.64, 389.95, 389.43, 389.43, 389.93, 389.49, 389.52, 390.05, 390.2, 390.22, 389.73, 389.71, 389.71, 390.06, 390.07, 389.96, 389.25, 389.16, 389.24, 389.19, 389.38, 389.46, 390.3, 390.54, 390.24, 390.25, 390.43, 390.04, 389.81, 389.61, 389.57, 389.7, 390.46, 390.54, 390.52, 390.63, 390.55, 391.28, 391.41, 393.07, 392.0, 391.16, 391.1, 391.08, 390.98, 390.72, 390.05, 390.55, 393.14, 391.94, 391.15, 391.03, 390.84, 391.22, 391.31, 391.5, 391.68, 392.07, 392.1, 392.06, 391.94, 391.76, 392.6, 392.6, 392.67, 391.72, 391.63, 391.24, 391.56, 392.06, 391.53, 391.51, 391.32, 391.41, 391.64, 391.9, 392.02, 391.91, 392.0, 391.76, 391.75, 391.7, 392.1, 392.25, 392.6, 392.76, 392.73, 392.78, 392.01, 391.48, 391.33, 391.89, 391.6, 391.7, 391.41, 391.51, 391.53, 391.54, 392.45, 392.65, 392.45, 392.79, 392.65, 392.77, 392.23, 392.24, 392.16, 392.55, 393.24, 395.25, 394.64, 394.49, 394.72, 393.2, 393.3, 393.49, 393.34, 393.46, 393.82, 393.46, 393.6, 393.6, 391.46, 392.38, 392.06, 392.59, 393.57, 393.23, 393.22, 393.87, 393.64, 394.17, 393.4, 393.29, 393.05, 392.37, 394.19, 394.2, 393.64, 393.2, 392.91, 395.48, 394.76, 392.52, 392.6, 392.47, 392.55, 392.64, 392.79, 393.82, 394.07, 394.26, 394.93, 394.72, 394.86, 394.96, 395.28, 395.62, 395.14, 395.27, 395.09, 394.96, 395.35, 395.11, 394.19, 394.09, 394.15, 393.92, 393.98, 394.38, 394.31, 394.02, 393.36, 393.41, 393.35, 393.44, 393.52, 393.58, 393.63, 393.25, 394.31, 394.37, 394.2, 393.98, 393.74, 392.9, 392.9, 393.58, 394.01, 393.91, 393.81, 392.83, 393.27, 393.47, 393.1, 393.41, 393.45, 393.49, 393.31, 393.46, 394.13, 393.53, 393.56, 393.63, 393.63, 393.48, 392.67, 392.02, 391.65, 391.06, 390.94, 390.88, 391.12, 391.88, 391.9, 392.18, 391.71, 392.32, 391.89, 391.67, 391.07, 391.12, 390.91, 390.84, 390.69, 390.45, 390.71, 390.59, 390.22, 390.78, 390.31, 389.38, 389.83, 390.3, 389.97, 389.64, 389.62, 389.66, 389.37, 389.27, 389.48, 389.72, 389.65, 389.68, 389.21, 388.28, 389.11, 389.03, 389.46, 389.03, 389.25, 389.31, 389.08, 388.96, 389.0, 389.45, 389.3, 389.37, 389.15, 389.32, 389.24, 388.9, 388.71, 388.6, 388.64, 388.9, 388.92, 388.97, 389.01, 389.01, 388.84, 388.94, 388.75, 388.69, 388.76, 388.77, 388.78, 388.97, 388.72, 388.49, 388.72, 389.02, 389.16, 389.04, 389.01, 389.29, 389.09, 389.04, 389.02, 389.33, 389.42, 389.1, 389.38, 389.21, 389.52, 389.53, 389.57, 389.55, 389.77, 389.75, 389.68, 389.65, 389.56, 389.59, 389.75, 389.92, 390.01, 389.81, 390.41, 390.28, 390.6, 390.65, 390.44, 390.34, 390.44, 390.62, 390.52, 390.49, 390.61, 390.46, 390.61, 390.53, 390.6, 391.41, 391.05, 391.15, 391.33, 390.9, 390.9, 391.01, 391.03, 391.37, 391.44, 391.42, 391.55, 392.0, 391.73, 392.37, 391.57, 391.69, 392.03, 392.16, 392.53, 392.17, 392.7, 392.19, 391.81, 392.06, 391.98, 391.96, 392.8, 392.26, 392.0, 392.25, 392.1, 392.13, 392.07, 392.74, 392.93, 393.4, 393.66, 393.37, 392.78, 392.29, 392.44, 394.24, 393.37, 395.24, 394.99, 391.96, 392.21, 392.5, 392.82, 392.99, 393.33, 392.84, 393.61, 393.61, 393.76, 393.21, 392.96, 392.95, 392.8, 392.87, 393.76, 392.18, 392.24, 392.15, 392.1, 395.48, 392.86, 392.93, 392.85, 393.44, 393.54, 393.94, 393.85, 394.82, 392.99, 393.85, 394.22, 394.26, 394.62, 394.6, 394.54, 394.11, 393.33, 393.34, 393.29, 393.32, 393.21, 393.3, 393.97, 393.71, 394.97, 395.17, 395.17, 394.93, 394.43, 395.09, 394.32, 394.43, 394.72, 394.61, 394.88, 395.17, 395.05, 395.02, 395.18, 396.26, 395.91, 395.71, 396.31, 397.16, 397.84, 397.76, 396.12, 396.35, 395.86, 396.23, 395.96, 396.24, 396.43, 396.8, 396.97, 397.16, 396.93, 396.76, 396.58, 396.72, 396.66, 396.88, 396.91, 396.98, 396.8, 397.59, 397.04, 397.19, 397.1, 397.22, 397.22, 397.3, 397.29, 397.39, 397.55, 397.57, 397.32, 397.15, 397.39, 397.47, 397.11, 395.83, 395.53, 396.41, 396.82, 396.85, 395.29, 396.45, 396.68, 396.5, 396.47, 395.25, 396.85, 396.73, 395.67, 396.9, 395.82, 395.61, 395.81, 395.24, 395.09, 396.88, 396.92, 396.69, 396.75, 396.28, 396.29, 394.93, 395.67, 395.84, 395.9, 396.11, 395.82, 395.9, 395.64, 395.8, 395.74, 395.71, 395.76, 395.52, 395.41, 395.3, 395.07, 395.18, 395.02, 394.74, 394.86, 394.77, 394.52, 394.66, 394.28, 394.97, 394.91, 394.89, 394.66, 394.14, 394.66, 393.94, 394.31, 394.18, 393.82, 394.07, 393.21, 393.12, 393.2, 393.6, 393.82, 393.23, 393.39, 393.59, 393.15, 393.47, 393.51, 393.1, 393.05, 392.44, 392.31, 392.57, 392.62, 392.62, 392.58, 392.28, 391.6, 391.45, 391.48, 391.77, 391.96, 391.78, 391.98, 391.99, 392.01, 392.01, 392.01, 391.9, 392.15, 392.11, 392.14, 391.84, 391.94, 390.3, 390.24, 391.45, 391.67, 391.41, 390.99, 390.53, 390.57, 391.01, 391.46, 391.13, 390.85, 390.99, 391.08, 391.06, 391.2, 391.54, 391.3, 391.31, 391.46, 391.59, 391.84, 391.41, 391.31, 391.52, 391.62, 391.46, 391.54, 392.15, 391.82, 391.76, 391.9, 392.63, 392.57, 392.49, 393.37, 392.81, 392.92, 393.12, 393.42, 393.6, 393.92, 393.74, 393.34, 393.18, 393.28, 393.35, 393.35, 393.45, 393.21, 392.94, 393.26, 394.54, 394.17, 393.57, 393.98, 394.02, 393.51, 393.39, 393.2, 393.44, 393.84, 393.79, 394.44, 394.3, 394.98, 394.18, 394.42, 394.7, 394.87, 394.56, 394.44, 394.64, 395.24, 395.36, 394.51, 394.69, 395.2, 395.03, 394.38, 394.78, 395.02, 394.97, 394.45, 394.57, 394.41, 394.71, 394.67, 394.94, 394.91, 395.4, 395.3, 395.26, 395.66, 395.7, 395.5, 395.7, 395.66, 395.65, 395.73, 396.84, 395.78, 395.66, 398.15, 396.73, 395.43, 395.49, 395.75, 395.95, 395.69, 395.48, 395.31, 395.44, 396.49, 396.32, 396.15, 395.66, 396.81, 396.7, 397.22, 396.58, 396.59, 396.68, 396.58, 396.87, 396.02, 396.16, 397.45, 398.08, 397.67, 397.54, 397.55, 396.65, 396.7, 397.26, 397.26, 396.84, 396.94, 396.94, 396.89, 398.53, 396.6, 396.56, 397.33, 396.68, 396.39, 396.81, 397.32, 397.39, 397.49, 397.5, 396.89, 397.46, 397.41, 397.37, 397.32, 397.23, 397.36, 397.12, 398.46, 398.35, 398.48, 398.62, 398.46, 398.71, 398.35, 398.41, 397.83, 397.76, 398.27, 398.45, 397.36, 397.7, 397.83, 397.34, 397.37, 399.41, 398.82, 399.06, 398.47, 398.54, 398.41, 399.72, 399.8, 399.6, 399.6, 399.49, 399.72, 399.98, 399.79, 399.59, 399.7, 399.87, 399.95, 399.57, 399.62, 399.59, 400.34, 400.08, 399.9, 400.43, 400.23, 399.98, 400.33, 399.9, 400.09, 400.03, 400.05, 400.26, 400.38, 400.71, 400.45, 400.23, 400.51, 398.59, 399.56, 399.61, 399.61, 399.23, 399.69, 399.51, 398.98, 398.81, 397.99, 399.15, 399.13, 398.99, 398.82, 398.41, 398.03, 398.11, 398.25, 398.12, 398.18, 398.54, 398.74, 398.94, 399.28, 399.23, 398.7, 398.77, 398.79, 398.69, 398.66, 398.27, 397.06, 397.44, 397.94, 398.11, 398.01, 397.67, 397.43, 397.86, 397.38, 397.05, 396.95, 397.06, 396.27, 397.4, 396.93, 397.1, 397.41, 397.45, 397.27, 396.18, 396.57, 396.95, 396.94, 397.02, 396.9, 395.76, 395.75, 395.84, 395.91, 395.62, 395.33, 395.35, 395.32, 395.38, 394.81, 394.9, 395.37, 395.43, 396.04, 394.42, 395.02, 395.37, 394.69, 394.61, 394.76, 394.83, 394.83, 393.74, 393.68, 394.71, 394.57, 394.62, 394.75, 393.31, 393.33, 393.5, 393.57, 393.95, 393.77, 393.77, 392.97, 393.3, 393.22, 393.37, 393.78, 393.77, 393.69, 393.62, 393.77, 393.7, 393.31, 393.43, 393.22, 393.5, 393.32, 393.24, 393.22, 393.54, 393.57, 393.45, 393.65, 393.36, 393.68, 393.56, 393.44, 393.55, 393.51, 393.58, 393.58, 393.84, 393.72, 393.77, 394.01, 393.98, 394.01, 393.93, 394.28, 394.12, 393.96, 393.97, 394.33, 394.35, 394.31, 394.23, 394.35, 394.33, 394.41, 394.39, 394.47, 394.48, 394.6, 394.59, 394.63, 394.63, 394.76, 394.8, 395.5, 396.67, 396.32, 395.64, 395.08, 394.79, 394.72, 394.75, 394.77, 394.74, 395.13, 394.96, 395.34, 395.83, 396.19, 396.31, 396.4, 395.76, 395.75, 396.09, 396.23, 396.32, 396.43, 396.43, 396.42, 396.45, 396.71, 396.61, 396.06, 395.97, 396.26, 396.62, 396.49, 396.42, 396.55, 396.5, 397.03, 397.78, 397.43, 397.15, 397.24, 397.31, 397.83, 397.64, 397.48, 397.58, 397.68, 397.75, 397.75, 397.42, 397.2, 397.74, 398.03, 398.41, 398.41, 398.65, 398.78, 398.55, 397.79, 397.48, 397.51, 397.53, 397.69, 397.5, 397.59, 398.41, 398.7, 398.05, 398.76, 398.44, 398.06, 398.0, 397.52, 397.93, 397.6, 397.74, 398.0, 398.16, 398.18, 397.96, 398.05, 397.99, 398.1, 398.26, 398.06, 398.04, 398.11, 398.18, 398.01, 397.95, 398.06, 397.8, 397.84, 398.26, 397.56, 397.38, 397.84, 398.39, 398.11, 398.52, 398.49, 398.61, 399.36, 397.64, 397.53, 397.57, 397.32, 397.64, 398.4, 398.68, 398.64, 398.7, 398.85, 398.87, 399.37, 399.4, 401.71, 400.3, 400.07, 399.82, 401.46, 401.3, 399.04, 399.97, 400.14, 400.09, 400.21, 400.33, 400.87, 400.68, 400.66, 400.9, 400.93, 401.4, 402.11, 402.3, 401.69, 401.53, 400.92, 400.7, 400.59, 402.26, 401.95, 401.31, 401.24, 401.27, 401.25, 401.98, 401.71, 401.59, 401.47, 401.67, 401.59, 401.3, 401.38, 401.54, 402.07, 401.92, 401.66, 401.67, 401.24, 402.32, 402.36, 402.47, 402.01, 401.9, 401.56, 401.33, 401.31, 401.43, 401.71, 402.01, 402.01, 402.67, 402.73, 401.98, 402.16, 402.03, 402.1, 401.75, 401.45, 401.4, 401.59, 401.63, 401.72, 401.58, 401.51, 401.15, 401.11, 401.47, 401.43, 401.4, 400.8, 401.33, 401.55, 401.24, 400.88, 400.55, 400.54, 400.83, 400.58, 400.5, 400.25, 399.9, 400.01, 400.22, 400.23, 399.88, 399.27, 399.23, 399.23, 399.23, 398.77, 398.07, 398.47, 398.95, 399.31, 399.12, 396.11, 396.25, 397.82, 398.04, 398.13, 397.8, 397.72, 397.37, 397.55, 397.16, 397.31, 397.26, 396.76, 396.83, 396.92, 396.33, 396.2, 396.58, 396.82, 396.35, 396.24, 396.64, 394.5, 394.56, 395.03, 395.0, 395.33, 394.66, 394.74, 395.35, 395.69, 395.72, 396.27, 395.8, 395.5, 395.91, 395.4, 395.57, 395.62, 395.61, 395.13, 395.67, 395.92, 396.03, 395.34, 395.45, 395.45, 395.51, 395.6, 395.87, 395.79, 395.75, 395.65, 395.87, 396.1, 395.99, 395.64, 396.26, 397.03, 396.39, 396.4, 397.11, 397.04, 396.77, 397.16, 397.03, 396.94, 396.9, 396.9, 397.76, 397.23, 397.55, 397.4, 397.7, 397.47, 397.69, 397.6, 397.02, 397.1, 397.28, 397.28, 398.8, 398.11, 397.92, 397.91, 397.68, 398.77, 398.35, 398.32, 398.23, 398.31, 398.56, 398.76, 398.76, 398.61, 398.82, 399.22, 399.49, 399.45, 399.38, 399.2, 398.69, 399.56, 398.9, 399.17, 399.42, 399.33, 399.28, 398.56, 398.69, 399.22, 399.56, 400.49, 399.94, 399.74, 399.9, 400.12, 400.18, 400.21, 399.69, 399.72, 399.83, 400.12, 400.23, 399.81, 399.55, 399.54, 399.65, 399.62, 399.8, 400.11, 400.87, 400.02, 400.01, 399.98, 400.06, 400.67, 400.54, 400.26, 400.25, 400.23, 400.09, 399.81, 401.11, 400.5, 399.56, 399.82, 399.84, 400.94, 400.05, 400.2, 399.93, 400.3, 400.14, 400.06, 399.86, 399.57, 399.88, 400.74, 400.98, 400.99, 401.11, 401.7, 401.28, 401.42, 401.04, 401.25, 401.53, 401.78, 401.79, 401.98, 401.93, 401.38, 401.37, 401.99, 402.61, 403.18, 402.14, 400.51, 400.46, 400.22, 400.04, 401.03, 401.86, 401.33, 401.17, 400.96, 402.63, 402.73, 403.36, 403.26, 400.96, 401.39, 402.53, 403.72, 404.45, 404.74, 403.21, 403.72, 403.42, 403.89, 403.85, 404.92, 404.76, 404.25, 404.37, 404.06, 403.62, 403.72, 403.66, 403.48, 403.92, 402.28, 402.42, 402.68, 403.62, 404.07, 403.81, 403.84, 403.88, 404.25, 403.63, 403.94, 404.23, 404.28, 404.44, 404.31, 404.09, 403.69, 403.84, 403.91, 403.86, 404.14, 404.25, 404.51, 404.34, 404.15, 404.05, 404.23, 404.12, 403.85, 403.92, 403.91, 403.71, 403.64, 403.34, 403.69, 404.15, 404.06, 403.84, 403.78, 403.46, 403.23, 403.24, 403.12, 402.96, 403.33, 403.37, 403.86, 403.77, 402.62, 402.56, 402.79, 402.91, 403.11, 402.55, 402.02, 401.97, 402.02, 402.01, 402.61, 403.02, 403.26, 403.01, 402.83, 403.09, 401.82, 401.59, 402.1, 402.29, 402.31, 401.98, 402.1, 402.08, 402.09, 402.71, 402.11, 401.41, 401.84, 401.89, 401.44, 401.15, 402.13, 401.19, 401.04, 400.61, 401.14, 401.21, 401.37, 401.35, 401.21, 401.07, 399.88, 399.91, 399.87, 397.02, 397.14, 398.19, 398.65, 398.74, 399.17, 399.34, 399.39, 399.54, 399.15, 399.16, 399.07, 398.79, 399.05, 399.21, 399.17, 398.91, 398.59, 398.26, 399.01, 399.24, 399.25, 399.28, 399.08, 398.5, 398.53, 398.97, 398.4, 398.83, 398.94, 398.91, 398.5, 397.9, 398.26, 398.07, 397.84, 398.29, 397.74, 397.66, 397.99, 397.43, 397.51, 396.15, 396.29, 396.16, 397.83, 397.42, 397.49, 397.37, 397.56, 397.31, 397.42, 397.3, 397.75, 397.37, 396.91, 397.2, 396.97, 397.88, 398.1, 398.27, 397.58, 398.83, 399.23, 399.17, 398.19, 398.3, 397.43, 397.92, 398.81, 398.37, 398.49, 398.47, 398.69, 398.4, 398.53, 397.78, 398.4, 398.65, 398.49, 398.52, 398.19, 398.52, 398.59, 398.92, 398.78, 398.55, 398.67, 399.07, 399.05, 399.23, 399.33, 399.28, 399.26, 399.58, 400.08, 399.49, 399.61, 401.58, 402.23, 400.28, 400.56, 400.28, 400.24, 400.55, 400.76, 400.35, 400.14, 400.17, 400.05, 400.46, 400.87, 400.62, 400.07, 401.31, 401.35, 400.68, 400.4, 400.46, 400.46, 400.47, 401.19, 401.18, 400.94, 401.2, 401.56, 401.26, 402.42, 402.94, 402.82, 402.84, 402.49, 402.21, 402.0, 402.02, 402.16, 402.85, 402.24, 402.35, 402.18, 401.83, 401.93, 401.71, 401.8, 401.81, 402.92, 402.13, 401.96, 401.81, 402.52, 401.96, 401.93, 401.99, 402.49, 402.32, 402.02, 402.03, 402.1, 403.5, 402.07, 402.57, 402.71, 402.5, 402.34, 402.68, 403.05, 402.76, 402.77, 403.04, 403.94, 403.78, 403.61, 402.56, 402.32, 402.51, 402.5, 403.09, 405.78, 405.62, 405.85, 405.1, 404.64, 404.37, 402.92, 402.62, 402.91, 403.07, 403.84, 403.54, 403.22, 403.21, 403.81, 404.05, 404.62, 405.85, 405.16, 404.89, 404.21, 403.74, 403.67, 403.65, 403.56, 404.72, 405.95, 404.24, 403.59, 403.39, 403.48, 403.67, 403.43, 403.93, 404.5, 404.78, 404.85, 404.51, 404.63, 403.9, 403.99, 405.01, 405.27, 405.22, 405.19, 405.8, 406.17, 406.02, 405.82, 406.46, 406.26, 406.16, 405.08, 404.6, 405.36, 406.03, 406.25, 405.89, 407.19, 406.91, 409.33, 409.38, 409.22, 408.89, 408.43, 408.82, 408.09, 408.05, 407.74, 407.72, 407.73, 407.07, 408.25, 407.97, 407.04, 406.61, 408.15, 408.04, 408.44, 407.76, 407.67, 407.73, 407.31, 407.41, 407.64, 407.4, 407.16, 407.72, 407.89, 408.15, 407.78, 407.37, 406.96, 407.11, 407.16, 406.87, 407.77, 407.72, 407.05, 407.95, 407.84, 408.14, 408.1, 407.73, 407.43, 407.18, 407.59, 407.13, 407.47, 407.65, 407.8, 407.38, 405.79, 407.23, 407.4, 407.06, 407.2, 406.88, 407.5, 407.13, 406.87, 406.9, 406.89, 406.75, 406.6, 406.62, 407.25, 406.08, 406.11, 406.18, 405.78, 406.05, 406.14, 406.3, 406.04, 405.77, 405.69, 405.32, 404.97, 405.3, 404.78, 404.73, 404.58, 404.32, 404.05, 404.24, 404.38, 403.35, 400.91, 403.11, 404.59, 404.08, 402.61, 403.48, 403.99, 403.71, 403.73, 403.87, 404.18, 403.95, 403.24, 403.18, 403.25, 403.56, 403.54, 403.26, 402.32, 403.2, 402.97, 403.04, 402.65, 401.84, 402.08, 401.39, 401.29, 402.61, 402.43, 400.84, 400.35, 402.03, 401.73, 401.95, 401.91, 401.85, 402.07, 401.42, 399.65, 401.08, 401.75, 400.99, 401.08, 401.42, 400.33, 400.42, 400.27, 401.5, 401.49, 401.68, 400.93, 401.0, 401.19, 401.73, 401.5, 400.95, 400.6, 401.12, 401.04, 400.59, 400.61, 400.76, 400.88, 400.44, 400.44, 400.94, 400.88, 400.43, 400.9, 400.91, 400.72, 400.49, 400.87, 400.74, 401.79, 401.56, 401.44, 401.56, 401.26, 401.74, 401.78, 401.8, 401.56, 401.73, 401.42, 401.55, 401.93, 401.7, 401.96, 402.07, 401.67, 401.63, 402.48, 402.87, 402.37, 402.07, 402.28, 403.06, 403.56, 403.42, 402.78, 402.84, 402.83, 403.01, 403.48, 402.16, 402.27, 402.62, 402.73, 405.01, 404.52, 403.97, 404.22, 403.88, 403.49, 403.67, 403.5, 403.28, 403.56, 405.45, 405.25, 404.84, 404.35, 403.87, 404.55, 404.55, 404.37, 403.99, 403.65, 403.4, 403.41, 403.91, 403.96, 404.24, 404.39, 404.4, 405.18, 404.83, 405.11, 405.0, 404.71, 404.18, 403.83, 404.3, 404.6, 404.94, 404.64, 404.6, 404.59, 404.44, 404.33, 404.82, 404.9, 404.97, 405.07, 406.86, 405.18, 405.37, 405.18, 405.68, 405.84, 405.9, 406.63, 405.83, 406.04, 406.03, 404.83, 406.32, 405.6, 405.68, 406.23, 406.07, 406.0, 406.88, 405.85, 406.46, 406.47, 405.77, 406.7, 407.97, 406.06, 405.48, 405.55, 405.75, 406.89, 406.28, 405.62, 406.31, 406.29, 406.41, 406.16, 405.97, 406.17, 405.82, 405.63, 405.62, 405.98, 406.51, 406.14, 405.74, 405.85, 405.64, 405.8, 405.74, 406.8, 407.14, 407.6, 407.77, 408.42, 408.29, 408.9, 407.41, 407.56, 406.67, 407.44, 407.52, 406.65, 406.67, 405.93, 405.61, 406.05, 407.44, 407.15, 406.47, 406.84, 406.58, 406.3, 406.65, 407.17, 406.81, 407.75, 406.85, 407.29, 409.48, 409.49, 409.17, 409.33, 408.56, 408.8, 408.19, 407.98, 408.18, 406.93, 406.94, 406.95, 407.71, 407.71, 407.88, 409.27, 409.09, 409.46, 409.71, 410.21, 410.2, 409.66, 409.71, 409.44, 409.72, 410.0, 410.0, 409.2, 409.5, 409.73, 408.6, 408.44, 408.45, 408.08, 410.49, 410.07, 409.6, 409.2, 409.55, 409.3, 409.19, 409.84, 409.34, 409.59, 410.51, 410.11, 410.18, 410.28, 410.21, 409.94, 410.01, 410.07, 410.05, 410.18, 410.03, 409.81, 409.94, 409.84, 409.58, 409.32, 409.49, 409.13, 409.52, 409.59, 409.75, 409.82, 409.88, 409.68, 409.49, 409.57, 409.15, 409.64, 409.7, 409.59, 409.52, 409.29, 408.73, 408.13, 408.11, 408.29, 408.22, 408.23, 408.49, 408.64, 408.4, 408.22, 407.53, 406.42, 406.74, 406.71, 409.61, 409.14, 408.99, 407.43, 407.3, 407.42, 407.33, 407.19, 406.73, 406.81, 406.91, 407.23, 406.83, 407.56, 407.52, 406.91, 406.92, 406.46, 406.59, 407.17, 406.39, 406.44, 406.68, 407.0, 406.98, 406.85, 407.03, 406.65, 403.89, 406.32, 406.35, 406.09, 405.69, 406.33, 404.69, 405.6, 405.99, 405.2, 405.29, 405.66, 405.58, 405.39, 405.16, 405.89, 405.68, 404.4, 404.52, 404.45, 404.24, 404.45, 404.86, 404.58, 404.75, 404.68, 403.96, 404.03, 403.99, 404.3, 404.24, 404.03, 404.06, 404.0, 403.68, 403.36, 403.54, 403.08, 403.15, 403.0, 403.53, 403.57, 403.76, 403.74, 403.72, 403.1, 403.35, 403.2, 402.73, 403.19, 402.97, 402.96, 402.99, 402.83, 402.36, 402.26, 402.69, 403.25, 402.47, 402.57, 402.97, 402.87, 403.03, 403.21, 403.21, 403.19, 403.34, 403.35, 403.37, 403.63, 403.96, 403.75, 402.95, 403.78, 404.04, 404.42, 404.17, 403.78, 403.78, 403.92, 404.09, 404.38, 404.21, 404.27, 404.07, 404.07, 404.24, 404.46, 404.46, 404.25, 403.89, 404.29, 404.27, 403.98, 404.47, 404.59, 404.59, 404.94, 404.95, 405.48, 405.28, 405.64, 405.49, 405.9, 406.04, 406.14, 406.61, 406.61, 406.02, 406.23, 406.6, 406.49, 406.15, 406.78, 406.93, 407.36, 407.11, 406.86, 406.19, 405.98, 405.87, 405.83, 405.78, 406.1, 406.24, 406.82, 407.02, 406.46, 406.21, 406.19, 406.52, 407.03, 407.72, 407.16, 406.9, 406.8, 406.82, 407.56, 407.54, 407.42, 407.27, 407.01, 406.8, 406.59, 407.77, 408.47, 407.64, 407.09, 407.56, 407.74, 408.92, 408.1, 408.71, 407.54, 408.54, 407.66, 407.76, 407.78, 407.72, 407.82, 408.34, 408.04, 408.9, 408.38, 407.91, 408.18, 407.82, 407.82, 407.66, 408.2, 408.29, 407.81, 407.66, 407.35, 407.28, 407.44, 407.66, 408.91, 409.51, 408.66, 408.81, 408.62, 407.85, 408.19, 408.37, 408.48, 408.52, 408.32, 408.26, 408.43, 408.31, 408.33, 408.56, 408.74, 408.82, 408.99, 408.75, 408.56, 408.67, 408.76, 409.28, 409.29, 409.01, 409.23, 409.98, 409.99, 409.99, 409.93, 409.83, 408.32, 408.54, 408.33, 408.99, 408.26, 408.65, 408.81, 408.74, 409.38, 409.99, 409.62, 409.27, 409.28, 410.31, 409.67, 409.96, 409.89, 409.6, 409.91, 410.13, 409.32, 409.46, 409.15, 408.73, 408.85, 409.15, 409.5, 409.37, 409.43, 410.33, 409.54, 409.59, 410.29, 410.29, 410.43, 409.87, 410.22, 410.48, 410.31, 410.72, 411.33, 411.71, 412.36, 412.13, 411.35, 410.65, 411.18, 408.84, 409.14, 409.09, 409.3, 410.57, 410.21, 411.78, 411.66, 411.64, 412.52, 412.0, 412.07, 411.7, 411.52, 412.17, 411.93, 409.99, 410.07, 411.58, 411.59, 411.86, 411.84, 411.63, 411.87, 411.84, 411.55, 411.71, 411.57, 410.6, 410.64, 410.42, 411.08, 410.72, 411.04, 411.12, 411.54, 411.29, 411.35, 411.19, 410.78, 411.1, 410.96, 410.85, 410.73, 409.74, 409.32, 411.3, 410.66, 410.94, 410.71, 410.94, 410.4, 410.34, 409.91, 411.91, 411.49, 409.63, 408.9, 409.22, 409.63, 409.73, 409.32, 409.45, 409.17, 409.35, 408.15, 409.26, 409.49, 409.57, 409.52, 409.27, 408.36, 408.66, 408.3, 408.1, 408.26, 408.36, 408.83, 408.37, 408.69, 408.42, 408.13, 407.89, 407.56, 407.23, 406.99, 407.38, 407.22, 407.26, 407.23, 407.44, 407.18, 406.98, 407.21, 406.93, 406.75, 407.14, 407.07, 407.0, 407.07, 407.08, 406.5, 406.57, 406.77, 406.59, 406.4, 406.42, 406.53, 406.4, 406.23, 406.12, 405.52, 405.78, 405.7, 405.8, 405.92, 405.86, 405.0, 405.3, 405.63, 405.64, 405.0, 405.23, 405.5, 405.79, 405.58, 405.83, 405.71, 405.87, 405.53, 405.64, 405.95, 405.58, 405.42, 405.42, 405.02, 405.87, 405.45, 405.45, 405.69, 405.52, 405.56, 405.47, 405.72, 405.42, 405.45, 405.4, 405.54, 405.53, 405.51, 405.9, 406.08, 405.91, 405.86, 406.12, 405.93, 406.13, 405.96, 406.2, 406.65, 406.63, 406.97, 406.87, 406.45, 406.55, 406.14, 406.83, 405.93, 406.32, 406.46, 406.65, 406.6, 407.09, 407.26, 406.88, 406.36, 406.92, 406.99, 407.19, 407.61, 407.84, 409.14, 409.15, 409.24, 409.81, 408.01, 408.56, 410.06, 408.39, 408.25, 408.39, 408.46, 408.44, 408.47, 408.42, 408.48, 408.35, 408.4, 408.24, 408.94, 408.52, 408.41, 408.25, 408.27, 408.35, 409.65, 409.42, 409.59, 409.63, 409.24, 411.1, 409.69, 409.24, 408.61, 408.97, 410.75, 409.59, 409.59, 409.59, 409.48, 409.31, 409.28, 409.25, 408.74, 408.79, 408.84, 409.22, 409.69, 409.77, 410.06, 409.93, 410.03, 408.9, 411.02, 411.07, 410.78, 410.44, 409.18, 409.37, 409.95, 409.97, 412.44, 413.32, 413.78, 413.9, 410.41, 410.38, 410.19, 410.9, 411.34, 411.38, 411.29, 411.31, 410.5, 410.65, 410.62, 410.77, 411.9, 410.44, 411.16, 410.78, 411.47, 413.53, 413.09, 412.42, 411.8, 412.19, 412.34, 412.48, 411.98, 411.48, 411.17, 410.4, 410.72, 410.84, 410.54, 411.3, 411.7, 412.18, 412.51, 411.78, 412.07, 411.84, 411.61, 412.37, 413.39, 410.96, 411.41, 411.45, 412.28, 413.35, 412.2, 412.23, 412.35, 412.09, 412.21, 410.92, 413.81, 414.72, 412.83, 411.43, 410.03, 410.05, 411.21, 410.82, 410.73, 410.52, 412.4, 412.41, 411.69, 412.13, 413.16, 412.25, 412.28, 412.34, 412.38, 412.99, 412.88, 413.48, 413.66, 414.02, 413.83, 414.43, 413.65, 413.54, 414.47, 414.38, 414.39, 414.56, 414.57, 414.84, 414.74, 414.02, 413.22, 414.53, 414.54, 414.36, 414.42, 414.39, 414.25, 415.18, 415.33, 415.43, 415.19, 415.65, 415.38, 415.14, 414.95, 414.64, 414.8, 414.51, 414.88, 414.88, 414.9, 414.26, 414.56, 414.15, 414.23, 414.42, 414.45, 413.91, 414.71, 413.19, 414.14, 414.6, 414.75, 414.31, 414.33, 414.14, 413.89, 414.65, 413.0, 413.24, 414.25, 413.61, 414.14, 413.98, 413.54, 413.24, 413.17, 413.48, 413.78, 413.44, 412.42, 413.57, 413.66, 413.42, 413.45, 413.13, 413.27, 413.09, 413.04, 410.82, 411.56, 411.82, 412.0, 412.66, 412.54, 411.78, 411.86, 412.13, 411.35, 411.35, 411.05, 409.73, 409.22, 410.29, 410.42, 410.68, 410.53, 410.42, 410.33, 410.31, 410.92, 409.91, 410.15, 410.31, 410.55, 410.32, 410.49, 410.66, 410.05, 410.21, 409.98, 410.37, 410.33, 409.05, 409.44, 410.07, 409.38, 409.06, 409.08, 409.62, 409.51, 409.77, 409.55, 409.2, 408.78, 409.14, 409.38, 408.5, 408.51, 408.42, 409.18, 409.02, 408.75, 408.82, 408.48, 408.44, 408.26, 408.59, 408.6, 408.71, 408.43, 407.97, 408.36, 408.5, 408.62, 408.4, 408.49, 408.05, 408.02, 407.96, 408.51, 408.34, 408.1, 407.66, 407.65, 407.77, 407.95, 407.99, 408.25, 408.2, 408.35, 408.89, 408.81, 408.38, 408.42, 408.39, 408.64, 408.83, 408.82, 408.73, 408.57, 408.6, 408.81, 408.65, 408.93, 408.65, 408.68, 408.57, 408.6, 408.96, 409.18, 409.78, 411.4, 409.02, 409.33, 409.46, 410.34, 409.97, 410.04, 410.43, 410.17, 410.09, 409.66, 410.23, 410.37, 410.72, 408.45, 409.88, 409.78, 410.11, 410.01, 410.09, 410.46, 410.37, 410.61, 410.41, 410.37, 410.58, 410.54, 410.77, 410.62, 410.88, 410.9, 411.34, 411.24, 411.09, 411.55, 411.24, 411.35, 410.91, 411.05, 411.27, 411.43, 411.66, 411.64, 411.69, 411.74, 411.73, 411.8, 411.91, 411.86, 413.11, 412.58, 412.08, 411.48, 412.46, 412.37, 412.22, 413.2, 412.87, 413.8, 412.62, 413.2, 413.4, 413.2, 413.09, 413.2, 413.57, 413.37, 413.39, 413.13, 413.16, 413.19, 412.51, 412.44, 412.93, 414.69, 414.24, 413.95, 414.04, 414.32, 414.39, 413.31, 413.59, 414.21, 414.02, 414.47, 414.98, 415.6, 415.31, 414.8, 413.22, 412.9, 413.55, 414.51, 413.55, 413.53, 414.59, 414.56, 414.33, 414.14, 414.17, 413.99, 413.17, 413.27, 413.48, 414.22, 414.18, 414.1, 413.88, 413.91, 413.13, 413.7, 414.42, 413.88, 414.4, 414.42, 414.12, 414.21, 414.2, 414.16, 414.21, 414.19, 414.53, 413.7, 414.22, 415.47, 415.56, 415.58, 415.63, 415.67, 415.72, 415.35, 414.96, 415.83, 415.69, 415.59, 415.64, 417.83, 416.87, 416.68, 416.02, 415.54, 416.05, 415.92, 416.84, 416.98, 416.83, 416.55, 416.12, 416.29, 416.17, 415.42, 415.4, 415.9, 415.45, 416.33, 416.06, 415.77, 417.33, 417.52, 417.31, 416.37, 416.41, 416.95, 417.31, 417.02, 416.57, 416.75, 416.57, 416.69, 417.15, 416.81, 416.93, 417.12, 417.02, 416.5, 417.85, 417.8, 417.94, 417.67, 417.83, 417.19, 416.67, 416.4, 416.87, 417.84, 417.13, 416.48, 416.18, 416.45, 416.7, 416.28, 415.79, 415.85, 416.45, 416.59, 416.6, 416.77, 416.74, 416.09, 415.99, 415.62, 416.09, 416.23, 416.29, 416.19, 416.26, 416.41, 415.56, 416.0, 416.0, 415.39, 415.26, 415.22, 415.13, 414.79, 415.11, 415.1, 415.3, 415.29, 415.31, 415.5, 414.93, 413.34, 414.78, 415.01, 415.19, 414.06, 414.08, 413.8, 413.85, 413.62, 413.85, 413.71, 413.92, 413.15, 413.03, 413.57, 413.68, 413.55, 413.29, 413.4, 413.45, 413.67, 412.8, 412.76, 413.02, 412.99, 412.41, 412.86, 412.85, 412.81, 412.65, 412.54, 412.71, 412.52, 412.23, 412.19, 412.45, 411.75, 412.13, 412.26, 411.86, 411.41, 411.75, 411.8, 411.79, 411.53, 411.95, 411.77, 411.66, 411.21, 411.38, 411.5, 411.36, 411.16, 410.94, 411.08, 411.38, 411.61, 411.91, 411.21, 411.11, 410.98, 411.08, 411.18, 411.13, 410.59, 410.9, 410.85, 411.11, 411.05, 410.36, 411.07, 411.09, 411.01, 410.97, 410.99, 411.12, 411.05, 411.08, 410.82, 411.08, 411.11, 411.04, 411.21, 410.83, 411.07, 411.14, 411.17, 411.11, 411.11, 411.19, 411.71, 411.97, 411.01, 411.3, 411.64, 411.61, 411.51, 412.2, 412.08, 411.43, 411.17, 412.26, 412.3, 412.03, 412.17, 413.82, 413.79, 412.69, 412.57, 412.4, 412.48, 412.27, 412.16, 412.11, 412.36, 412.29, 414.33, 413.69, 413.2, 413.04, 413.23, 415.22, 415.65, 415.31, 413.77, 413.65, 413.55, 412.71, 412.78, 412.74, 412.78, 413.05, 413.39, 413.92, 413.57, 413.74, 413.57, 413.44, 413.33, 413.58, 414.08, 414.15, 414.26, 414.66, 414.59, 414.41, 414.22, 414.65, 414.58, 414.6, 414.5, 414.76, 414.61, 414.68, 415.09, 415.21, 415.37, 415.29, 415.02, 414.96, 414.85, 414.86, 414.73, 414.87, 414.87, 414.69, 414.8, 414.75, 414.73, 414.82, 415.18, 414.8, 414.95, 415.22, 415.22, 416.52, 415.58, 415.57, 414.55, 415.65, 415.59, 415.85, 415.84, 415.6, 415.52, 415.72, 415.64, 419.1, 418.15, 417.07, 416.15, 415.84, 416.39, 416.93, 417.14, 417.02, 416.42, 415.82, 416.02, 415.92, 415.73, 416.48, 416.68, 416.07, 415.85, 416.19, 416.04, 415.96, 416.14, 416.26, 417.19, 416.62, 418.48, 417.91, 418.03, 416.9, 417.37, 416.18, 416.26, 415.95, 416.12, 416.55, 417.07, 416.9, 416.83, 416.93, 418.19, 418.32, 417.38, 418.05, 418.82, 416.16, 416.12, 416.83, 417.17, 417.08, 418.29, 418.31, 418.27, 419.12, 419.09, 419.31, 419.32, 418.32, 417.97, 417.88, 418.0, 417.73, 416.46, 417.27, 418.16, 418.26, 417.94, 419.31, 419.66, 419.34, 419.28, 419.56, 420.29, 419.74, 419.4, 419.4, 419.8, 418.03, 418.34, 418.4, 417.51, 418.3, 418.41, 417.83, 418.76, 419.21, 419.12, 419.53, 419.64, 418.18, 417.84, 417.42, 417.76, 418.05, 418.61, 418.48, 419.55, 419.8, 419.6, 420.06, 419.95, 419.69, 418.84, 419.32, 419.45, 419.54, 419.34, 419.13, 418.9, 418.39, 418.71, 418.91, 418.57, 418.25, 418.83, 418.64, 418.84, 418.24, 417.88, 418.65, 417.48, 417.13, 417.53, 417.83, 418.49, 417.69, 418.25, 418.17, 417.45, 416.96, 417.94, 417.9, 417.35, 417.48, 417.58, 416.86, 416.7, 416.52, 417.32, 417.06, 417.05, 417.01, 416.72, 416.73, 416.67, 415.74, 415.55, 415.78, 415.93, 414.97, 415.05, 415.35, 415.36, 414.8, 414.38, 414.79, 415.01, 415.14, 415.08, 414.68, 414.47, 413.36, 414.68, 414.55, 414.54, 414.67, 414.82, 414.52, 415.01, 414.76, 414.86, 414.45, 414.51, 414.74, 414.14, 413.75, 413.92, 413.62, 411.63, 411.75, 409.65, 413.12, 413.14, 413.06, 413.05, 413.47, 413.43, 413.57, 413.01, 412.83, 413.15, 413.46, 412.99, 412.89, 412.94, 412.96, 413.3, 413.28, 413.31, 413.18, 413.1, 412.92, 412.89, 412.91, 413.02, 413.5, 412.41, 413.19, 413.18, 413.28, 413.11, 413.21, 413.65, 413.34, 413.93, 413.62, 413.62, 413.43, 413.47, 413.42, 413.42, 414.04, 414.34, 414.4, 414.16, 413.92, 414.15, 413.26, 414.12, 413.76, 413.96, 413.53, 413.64, 413.59, 413.66, 413.75, 413.25, 414.11, 414.09, 414.08, 414.05, 414.19, 414.53, 414.79, 414.72, 414.79, 415.33, 415.47, 414.5, 414.36, 414.39, 414.54, 414.5, 414.47, 415.07, 415.0, 414.97, 414.95, 415.09, 414.93, 415.2, 415.18, 415.3, 415.56, 416.32, 416.59, 415.74, 415.81, 415.68, 415.97, 415.56, 415.86, 415.64, 415.7, 415.7, 416.2, 416.69, 416.7, 416.62, 415.96, 416.39, 416.65, 416.81, 416.84, 417.99, 417.47, 417.06, 416.92, 417.06, 417.67, 417.17, 416.95, 416.86, 417.54, 417.52, 417.0, 417.11, 417.69, 417.24, 416.64, 417.13, 417.57, 417.67, 417.71, 417.99, 418.75, 418.82, 418.51, 417.59, 417.36, 417.11, 417.18, 417.49, 417.51, 417.1, 419.21, 419.65, 420.18, 420.27, 418.03, 417.99, 420.08, 418.96, 419.11, 419.07, 419.18, 419.03, 418.85, 418.47, 418.52, 418.35, 418.47, 417.71, 417.68, 417.43, 421.29, 420.06, 419.64, 418.8, 418.58, 418.46, 418.63, 418.49, 419.87, 420.15, 420.62, 419.59, 419.01, 419.32, 419.08, 419.04, 418.79, 418.65, 418.59, 418.53, 418.31, 417.74, 417.91, 418.19, 419.33, 419.22, 418.76, 418.43, 418.07, 417.8, 418.96, 417.62, 417.86, 417.75, 417.74, 417.72, 417.79, 417.88, 417.88, 419.51, 420.31, 419.75, 419.85, 419.62, 420.03, 420.07, 420.43, 420.32, 419.89, 419.0, 419.64, 420.39, 418.69, 418.6, 419.35, 420.48, 420.25, 420.21, 421.35, 420.57, 420.43, 420.66, 420.23, 420.25, 419.45, 419.39, 420.26, 421.14, 419.33, 419.27, 419.92, 420.36, 420.11, 420.09, 419.32, 418.91, 419.06, 419.39, 419.89, 420.99, 421.37, 421.57, 421.69, 421.49, 421.38, 421.41, 420.65, 419.6, 420.43, 420.1, 420.1, 421.01, 421.19, 421.48, 421.18, 421.14, 421.53, 421.41, 421.3, 421.27, 421.5, 421.54, 421.08, 421.18, 421.19, 421.2, 421.03, 421.09, 420.82, 420.87, 420.75, 420.71, 420.89, 421.2, 419.88, 420.29, 420.48, 420.83, 420.66, 420.44, 420.5, 420.47, 420.52, 420.46, 419.84, 419.74, 419.85, 420.18, 419.7, 420.2, 420.23, 420.13, 419.28, 419.82, 419.28, 419.27, 418.79, 418.82, 418.69, 418.79, 418.97, 418.76, 418.88, 418.98, 417.91, 418.66, 418.36, 417.97, 417.69, 418.1, 418.28, 418.34, 418.03, 417.1, 417.02, 417.8, 418.06, 418.08, 417.41, 418.15, 417.59, 417.43, 417.62, 416.79, 416.93, 416.97, 416.89, 416.36, 416.7, 417.15, 416.45, 417.25, 416.43, 415.92, 416.06, 416.5, 416.26, 416.3, 416.24, 415.78, 415.94, 416.1, 416.33, 416.35, 416.28, 414.0, 416.13, 415.41, 415.82, 415.67, 415.83, 415.96, 416.17, 415.67, 415.94, 415.53, 415.51, 415.74, 415.86, 415.97, 415.14, 414.33, 414.41, 415.33, 414.77, 414.93, 415.17, 415.37, 415.34, 415.53, 415.19, 414.68, 414.6, 414.57, 414.67, 415.0, 414.97, 414.97, 414.85, 415.0, 415.0, 415.13, 415.25, 415.55, 415.56, 415.3, 415.36, 415.42, 415.62, 415.68, 415.64, 415.66, 415.82, 415.33, 415.96, 416.68, 415.98, 415.82, 416.33, 415.83, 415.89, 416.06, 416.23, 416.28, 416.38, 416.22, 416.54, 416.98, 416.84, 417.52, 416.74, 416.71, 416.79, 416.75, 417.25, 417.83, 417.81, 418.53, 418.47, 418.58, 417.85, 417.59, 416.87, 416.98, 422.14, 422.36, 422.47, 421.9, 421.82, 421.91, 420.1, 420.17, 420.66, 422.0, 420.39, 420.49, 421.0, 420.97, 420.87, 420.69, 420.73, 420.68, 420.23, 419.91, 420.22, 420.37, 418.97, 418.99, 418.82, 419.16, 418.84, 418.5, 418.51, 418.31, 418.23, 418.4, 418.16, 418.51, 418.54, 417.34, 417.36, 418.12, 417.98, 418.07, 418.1, 418.01, 417.58, 417.96, 417.84, 417.47, 418.24, 418.22, 418.05, 418.12, 417.95, 418.2, 418.04, 418.4, 418.45, 419.35, 419.08, 419.19, 419.21, 418.63, 418.61, 418.61, 418.58, 418.66, 418.67, 418.53, 418.53, 418.73, 418.67, 419.28, 419.42, 419.07, 419.03, 418.96, 418.88, 419.0, 419.14, 419.15, 419.22, 421.09, 421.44, 421.88, 420.75, 420.64, 421.23, 421.2, 421.24, 420.58, 420.09, 420.5, 421.11, 421.32, 421.55, 421.69, 423.1, 423.09, 422.98, 422.46, 422.19, 421.59, 421.9, 422.3, 421.9, 421.19, 421.47, 422.07, 422.19, 422.38, 422.86, 423.15, 423.01, 422.9, 424.63, 423.92, 422.36, 422.23, 423.27, 422.94, 422.53, 422.68, 422.63, 422.81, 422.54, 422.4, 422.53, 422.28, 422.48, 422.37, 422.07, 422.04, 422.12, 422.04, 421.95, 422.01, 426.4, 425.63, 425.8, 425.12, 425.46, 425.7, 424.45, 423.51, 423.51, 422.54, 422.78, 423.01, 423.11, 424.96, 425.06, 425.08, 424.94, 424.82, 425.69, 424.95, 424.99, 424.47, 424.25, 424.28, 424.53, 424.79, 425.37, 425.96, 425.2, 424.13, 424.4, 427.8, 427.07, 424.78, 423.73, 426.47, 425.83, 425.28, 426.47, 426.16, 424.4, 424.57, 425.19, 425.92, 426.26, 425.73, 425.87, 425.77, 426.33, 427.21, 424.6, 424.51, 425.2, 424.86, 424.74, 426.87, 427.13, 427.07, 425.92, 426.37, 427.08, 427.05, 427.77, 428.08, 428.39, 428.63, 426.52, 425.57, 425.58]
# TEST
co2_concentration = extract_data(co2e_data, 'co2_concentration')
ch4_concentration = extract_data(co2e_data, 'ch4_concentration')
decimal_year = extract_data(co2e_data, 'decimal_year')
assert co2_concentration[0] == 350.84
assert ch4_concentration[0] == 1.70002
print('All tests passed!')
All tests passed!
Step 3 - Smoothing the data#
Before we do anything with the data it is a good idea to visualise it. We can do this using matplotlib. For example, the following code will plot the CO2 concentrations against time:
import matplotlib.pyplot as plt
plt.plot(decimal_year, co2_concentration)
Copy this into the cell below and run it to see the plot.
# SOLUTION
import matplotlib.pyplot as plt
plt.plot(decimal_year, co2_concentration)
[<matplotlib.lines.Line2D at 0x7fe24888a110>]
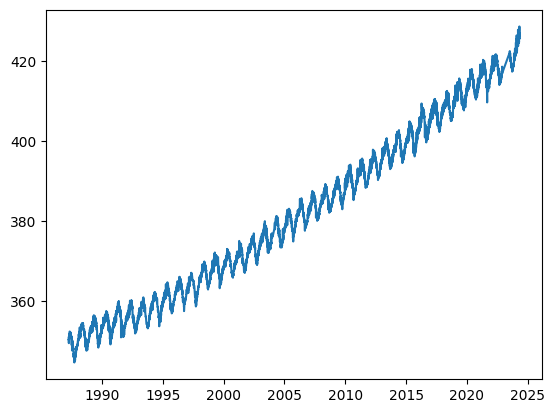
You should see a graph that trends upwards over time but which also has a season oscillation, i.e., the concentration of CO2 in the atmosphere increases and decreases over the course of a year. This is because the concentration of CO2 in the atmosphere is affected by the seasons. In the summer, plants grow and absorb CO2 from the atmosphere, and in the winter they die and release CO2 back into the atmosphere.
Let’s say that we now want to calculate the date and value of the maximum and minimum CO2 concentrations, i.e., the peaks and the dips in the graph. One way of finding a peak is to look for a value that is greater than the values either side of it. For example, if we have a list of values [1, 2, 3, 2, 1]
then the value 3 is a peak because it is greater than the values either side of it. However, if we apply this logic to the data directly we will find that there are many peaks and dips that are not of interest, i.e., if we zoom in on one year we will see that the data is very noisy.
In the cell below, repeat the plot but only using the first 300 elements of decimal_year
and co2_concentration
. By looking at just this small segment of the data, you should be able to see these unwanted peaks and dips more clearly.
# SOLUTION
plt.plot(decimal_year[0:300], co2_concentration[0:300])
[<matplotlib.lines.Line2D at 0x7fe2486dcbd0>]
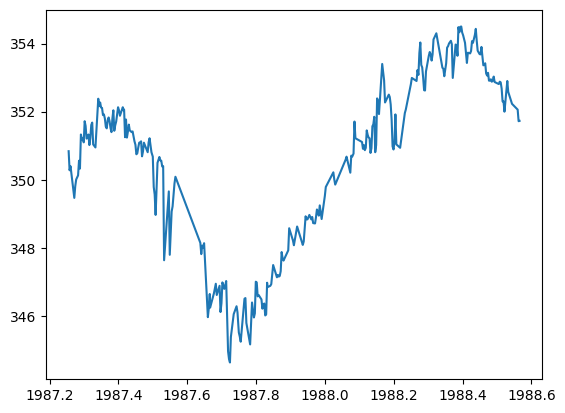
The solution to this is to apply a ‘smoothing filter’ to the data before we look for the picks and dips. A smoothing filter is a function that takes a list of numbers as input and returns a new list of numbers that are ‘smoothed’ versions of the input. It does this by sliding a window over the input list and calculating the weighted sum of the numbers in the window.
For example, say that we have a list of numbers [1, 2, 3, 2, 1, 2, 3, 1]
and we want to apply a smoothing filter with a window size of 3 with the window values [0.25, 0.5, 0.25]
. The first smoothed output value will be calculated by taking the first three numbers [1, 2, 3]
and computing the weighted sum 0.25*1 + 0.5*2 + 0.25*3 = 2
. We then step forward one place and take the numbers [2, 3, 2]
and compute the smoothed value as 0.25*2 + 0.5*3 + 0.25*2 = 2.25
, and so on.
Note, the weights in the window must sum to 1.0. This is because we want the smoothed values to be on the same scale as the input values. If the weights do not sum to one we can simply normalise them by dividing each weight by the sum of the weights.
Write a function called smooth_series(series, window)
that takes two parameters: series
- a list of numbers to smooth, and window
- a list of numbers to use as the weights. Remember to normalise the weights so that they sum to 1.0. The function should return a new list of smoothed values.
Write the function below and then check it by running the test cell.
# SOLUTION
def smooth_series(series, window):
y = []
N = len(window)
window_sum = sum(window)
for i in range(len(series)-N+1):
y.append(sum(x*w for x,w in zip(series[i:i+N], window)))
y = [yi/window_sum for yi in y]
return y
# TEST
series = [1, 2, 1, 2, 1]
assert smooth_series(series, [1]) == series
assert smooth_series(series, [1, 1]) == [1.5, 1.5, 1.5, 1.5]
print("All tests passed!")
All tests passed!
We will now use the smoothing function to smooth the data. The function allows the window to be of different lengths. The longer the window is, the smoother the data will be. If we make the window too long, then we will lose the annual peaks and dips that we are interested in (this is called ‘over-smoothing’).
Test the smoothing by using window lengths of 7, 60, 360 and plot the result. Store the smoothed data in variables called co2_concentration_smooth7
, co2_concentration_smooth_60
and co2_concentration_smooth360
respectively. Plot the smoothed data on the same graph as the original data to compare them.
# SOLUTION
import matplotlib.pyplot as plt
co2_concentration_smooth7 = smooth_series(co2_concentration, [1] * 7)
co2_concentration_smooth60 = smooth_series(co2_concentration, [1] * 60)
co2_concentration_smooth360 = smooth_series(co2_concentration, [1] * 360)
plt.plot(co2_concentration[1000:1600])
plt.plot(co2_concentration_smooth7[1000-3:1600-3])
plt.plot(co2_concentration_smooth60[1000-30:1600-30])
plt.plot(co2_concentration_smooth360[1000-180:1600-180])
plt.show()
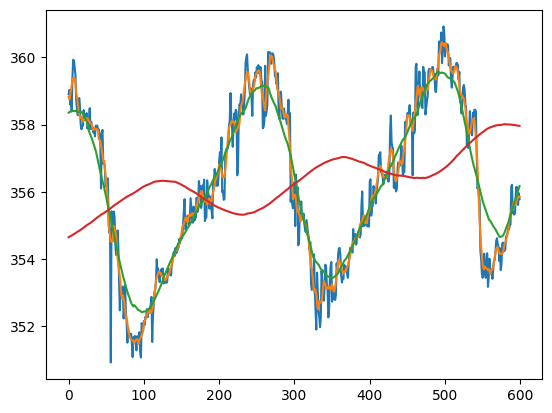
You should observe that the using a window of 7 is too short and the peaks and dips are still very noisy. A window of 360 is too long and the peaks and dips are no longer correct. The window of 60 is about right.
Step 4 - Finding the peaks#
We will now locate the peaks and dips in the smoothed data. We will look for peaks by looking for values that are greater than the values either side of them. We can also call these ‘local maxima’. We will write a function that takes a list of values and compares each value with its neighbours. If the value is greater than both of its neighbours then it is a peak and we will add the value to a list of peaks. We will also record the index of the peak in a list of peak indices. The function will return the list of peaks and the list of peak indices.
Write a function called find_peaks
that takes a list of values as input and returns a list of peak values and a list of peak indices. Test your function by running the test cell.
# SOLUTION
def find_peaks(series):
peaks = []
indices = []
for i in range(1, len(series)-1):
if series[i-1] < series[i] and series[i] > series[i+1]:
peaks.append(series[i])
indices.append(i)
return peaks, indices
# TEST
peaks, indices = find_peaks([1,2,1,1,1,2,1,1,2,1])
assert len(peaks) == 3
assert len(indices) == 3
assert indices == [1, 5, 8]
print("All tests passed!")
All tests passed!
Now, in the cell below, run the find_peaks
function on the smoothed CO2 concentration data. How many peaks are there? Does there seem to be about one per year (remember, the data spans a 35 year period). Are the indices of the peaks regularly spaced by about 365 days?
# SOLUTION
peaks, indices = find_peaks(co2_concentration_smooth60)
print(len(indices))
print(indices)
186
[9, 94, 221, 223, 231, 316, 319, 468, 475, 480, 559, 705, 713, 717, 725, 815, 976, 979, 983, 986, 1057, 1220, 1227, 1233, 1319, 1461, 1466, 1669, 1673, 1862, 1924, 1933, 2021, 2165, 2177, 2251, 2352, 2355, 2418, 2556, 2561, 2569, 2572, 2576, 2647, 2736, 2770, 2803, 2808, 2811, 2910, 3068, 3152, 3273, 3276, 3452, 3457, 3614, 3690, 3696, 3707, 3782, 3785, 3867, 3933, 3935, 3943, 4105, 4107, 4114, 4119, 4126, 4142, 4420, 4423, 4431, 4710, 4714, 4808, 4817, 4947, 4956, 4995, 5003, 5005, 5011, 5014, 5087, 5104, 5280, 5283, 5295, 5303, 5402, 5406, 5506, 5594, 5596, 5598, 5711, 5830, 5853, 5900, 5906, 5909, 5917, 5922, 5927, 5932, 5938, 6032, 6158, 6220, 6224, 6228, 6523, 6529, 6536, 6542, 6654, 6656, 6766, 6841, 6853, 6861, 6941, 6949, 7133, 7139, 7154, 7249, 7263, 7270, 7343, 7481, 7596, 7600, 7728, 7803, 7818, 7835, 7949, 7953, 8070, 8161, 8168, 8171, 8179, 8279, 8283, 8391, 8417, 8474, 8487, 8494, 8501, 8612, 8807, 8814, 8931, 9080, 9132, 9139, 9144, 9146, 9241, 9255, 9383, 9385, 9396, 9403, 9406, 9414, 9422, 9429, 9469, 9472, 9475, 9480, 9485, 9490, 9590, 9594, 9663, 9671, 9678]
You will find that there are still too many peaks because the data smoothing is not perfect. Rather then increasing the smoothing window length, we can fix things by using a more robust definition of ‘peak’. We will say that a peak needs to be larger than all surrounding values up to a distance of 2 steps away (i.e., the 3 in 1, 2, 3, 2, 1 would be a peak, but the 3 in 4, 2, 3, 2, 1 would not).
Implement this new definition of peak in a function called find_peaks2
and test it by running the test cell.
# SOLUTION
def find_peaks2(series):
peaks = []
indices = []
for i in range(3, len(series)-3):
if series[i-1] < series[i] and series[i] > series[i+1] and series[i] > series[i-2] and series[i] > series[i+2] and series[i] > series[i-3] and series[i] > series[i+3]:
peaks.append(series[i])
indices.append(i)
return peaks, indices
# TEST
peaks, indices = find_peaks2(co2_concentration_smooth60)
print(len(indices))
print(indices)
84
[9, 221, 468, 475, 480, 713, 725, 976, 1227, 1461, 1466, 1669, 1933, 2165, 2177, 2251, 2352, 2556, 2561, 2569, 2576, 2808, 3068, 3152, 3273, 3457, 3696, 3707, 3782, 3867, 4105, 4114, 4119, 4126, 4423, 4714, 4817, 4947, 5011, 5283, 5303, 5406, 5598, 5853, 5900, 5922, 5927, 5932, 5938, 6032, 6220, 6224, 6542, 6654, 6766, 6853, 7133, 7139, 7154, 7249, 7481, 7596, 7818, 7835, 7949, 8168, 8179, 8279, 8283, 8417, 8494, 8807, 8814, 9080, 9132, 9139, 9144, 9385, 9422, 9472, 9480, 9485, 9490, 9678]
You’ll see that there are still too many peaks, i.e. more than one per year. We can quickly see what is going on by plotting points at the peak positions overlaid on the original data. Run the cell below to see this.
plt.plot(co2_concentration_smooth60)
plt.plot(indices, peaks, 'ro')
plt.show()
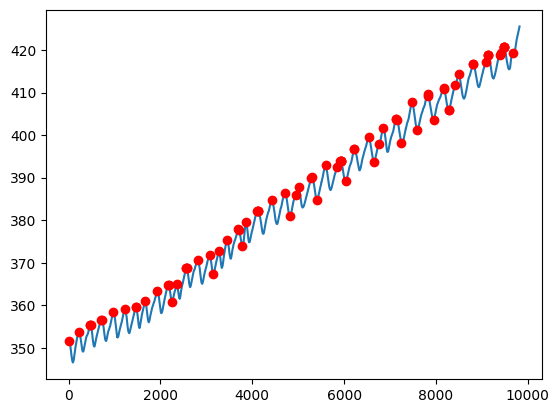
Observe the figure above. You should see several problems,
At least one of the genuine peaks has been missed.
One peak has been detected twice.
Strangely, there are many peaks being detected at the location of minima.
Peaks are located at the location of minima because the gradient of the curve is low at the minima, so a small amount of noise can raise a false peak, despite all the smoothing.
This exercise has illustrated how difficult it can be to extract something as simple as a peak from a noisy signal. The peaks are clear to the human eye, but it is difficult to write a program that can detect them reliably. We need more sophisticated statistical modelling approaches if we want to do this robustly.
You will have also noticed that we have had to write Python code for some very low level operations, such as smoothing and finding peaks. This is a lot of work and it is easy to make mistakes. Also, the code has involved using loops to process the values in the list. This is slow and inefficient. We will now see how NumPy can help us to solve these problems.
Step 5 - Finding the peaks with NumPy#
We will now repeat the exercise above using NumPy. We will start with the original data series co2_concentration
and decimal_year
.
Currently, the data are stored as a Python list. To turn them into NumPy arrays, we can use the NumPy.array function. For example, the following code will convert the list of co2_concentration
values into a NumPy array called co2_concentration_array
.
import numpy as np
co2_concentration = np.array(co2_concentrations)
Convert the co2_concentration
and decimal_year
lists into NumPy arrays and then plot the co2_concentration_array
against the decimal_year_array
. You should see the same graph as before.
# SOLUTION
import numpy as np
co2_concentration = np.array(co2_concentration)
decimal_year = np.array(decimal_year)
plt.plot(decimal_year, co2_concentration)
[<matplotlib.lines.Line2D at 0x7fe24850a110>]
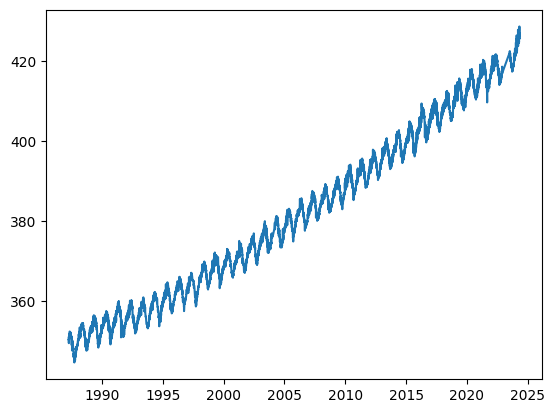
# TEST
assert type(co2_concentration) == np.ndarray
assert type(decimal_year) == np.ndarray
print("All tests passed!")
All tests passed!
We will now rewrite the smooth_series
function so that it takes the series and window parameters as NumPy arrays. The function should return a NumPy array of smoothed values. The function should work for any window size.
Note, you can use the fact the NumPy allows two vectors to be multiplied together in an element-wise fashion, e.g. x*y will multiply the first element of x by the first element of y, the second element of x by the second element of y, and so on. You can also make use of the numpy.sum
function to sum the elements of an array. You will still need to pass the window over the series using a loop and use slicing notation to extract segments of the series to operate on.
# SOLUTION
def smooth_series(series, window):
N = len(window)
smoothed = np.array([np.sum(series[i:i+N] * window) for i in range(len(series)-N+1)])
return smoothed / np.sum(window)
# TEST
series = np.array([1, 2, 1, 2, 1])
assert (smooth_series(series, np.array([1])) == series).all()
assert (smooth_series(series, np.array([1, 1])) == np.array([1.5, 1.5, 1.5, 1.5])).all()
print("All tests passed!")
All tests passed!
The NumPy implementation will have been a little shorter and will run faster. However, we can do much better. The operation of sliding a window over a single and multiplying and summing is known as a ‘convolution’. NumPy has a function called numpy.convolve
that will do this for us. The following code will apply a smoothing window to a series of values:
smoothed = np.convolve(series, window, mode='same')
This is actually better than our smoothing function because the mode='same'
parameter ensures that the function correctly pads the single to make sure the output and input have the same number of elements.
Try using the convolve function to smooth the co2_concentration_array
using a window of 90. Store the results in a variable called co2_concentration_smooth90
. Plot the result and compare it to the previous result. You should see that the results are the same. Note that when plotting, you will need to ignore the first and last 45 samples because the zero padding will mean that these will not have valid values.
Note that you can use the np.ones
function to make the window array. You will need to divide the window by the sum of the window values to make sure that the weights sum to 1.0 (the convolve
function does not do this for you).
# SOLUTION
co2_concentration_smooth90 = np.convolve(co2_concentration, np.ones(90)/90, mode='same')
plt.plot(decimal_year[45:-45], co2_concentration[45:-45])
plt.plot(decimal_year[45:-45], co2_concentration_smooth90[45:-45])
[<matplotlib.lines.Line2D at 0x7fe2483bfa50>]
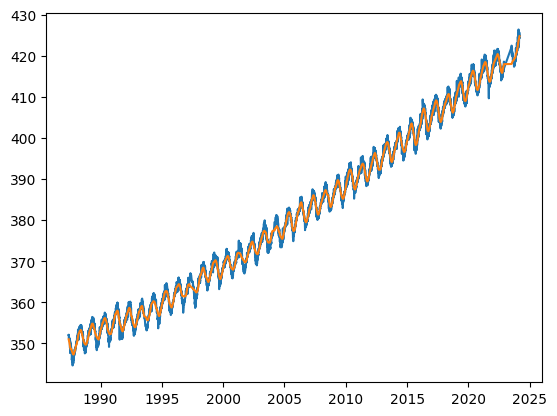
# TEST
assert co2_concentration.shape == co2_concentration_smooth90.shape
You will have noticed that the smoothed function consistently underestimates the height of the peaks. This is because it is based on an average over 90 days, and so the values before and after the peaks pull the average down. You can improve the situation by using a window function that has bigger weights at the centre of the window and that tapers to zero at the edges. There are many window shapes that can be used (e.g., a triangle), but a common one in signal processing is the Hamming window. The values for an N-element Hamming window can be produced with NumPy using the following code:
window = np.hamming(N)
Try using the np.convolve
function again but now use np.hamming
to generate the window. Remember, you will need to divide the window values by their sum so that it sums to 1.0.
Store the new smoothed data in a variable, co2_concentration_smooth90_h
.
To make the comparison, plot the co2_concentration
data, and the 90-day smoothed versions using both the window of ones and the Hamming window. To see the difference more clearly, just look at the first 500 samples of the signals. You should see that the peaks are now better estimated.
# SOLUTION
co2_concentration_smooth90_h = np.convolve(co2_concentration, np.hamming(90)/np.sum(np.hamming(90)), mode='same')
plt.plot(decimal_year[45:500], co2_concentration[45:500])
plt.plot(decimal_year[45:500], co2_concentration_smooth90[45:500])
plt.plot(decimal_year[45:500], co2_concentration_smooth90_h[45:500])
[<matplotlib.lines.Line2D at 0x7fe24844f9d0>]
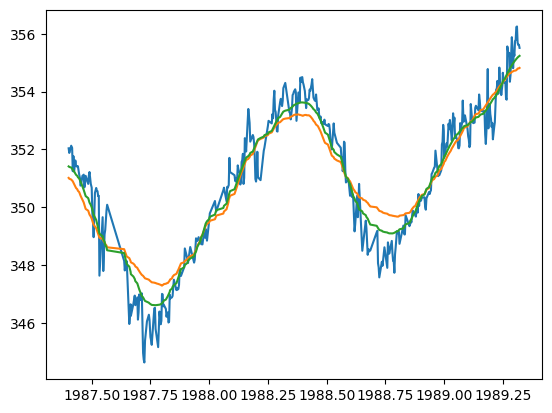
We will now rewrite the find_peaks2
function so that it takes a NumPy array as input and returns a NumPy array of peak values and a NumPy array of peak indices.
The function should be implemented without using any for-loops. Instead, you should use NumPy array operations to compare each element of the input array to its neighbours. There are many different ways to do this. The approach should be to build an array of logic values that has ‘True’ at the location of the peaks. You can then use the numpy.where
function to extract the indices of the peaks and the values of the peaks.
You might find it useful to use the numpy.roll
function to shift the array by one element. Once the array is shifted, you can compare the values in the original array to the shifted array. You can also effectively shift the array using slicing notation, e.g., consider the arrays series[4:]
, series[3:-1]
, series[2:-2]
, series[1:-3]
, series[:-4]
. These all have the same length, but are shifted by different amounts.
You can then use the numpy.logical_and
function to combine the results of the comparisons.
If you are very stuck then seek assistance from a demonstrator.
# SOLUTION
def find_peaks2(series):
peaks = (series[2:-2] > series[1:-3]) & (series[2:-2] > series[3:-1]) & (series[2:-2] > series[4:]) & (series[2:-2] > series[:-4])
indices = np.where(peaks == True)[0]
return series[indices], indices
# TEST
peaks, indices = find_peaks2(co2_concentration_smooth90_h)
plt.plot(decimal_year[45:-45],co2_concentration_smooth90_h[45:-45])
plt.plot(decimal_year[indices], peaks, 'ro')
plt.show()
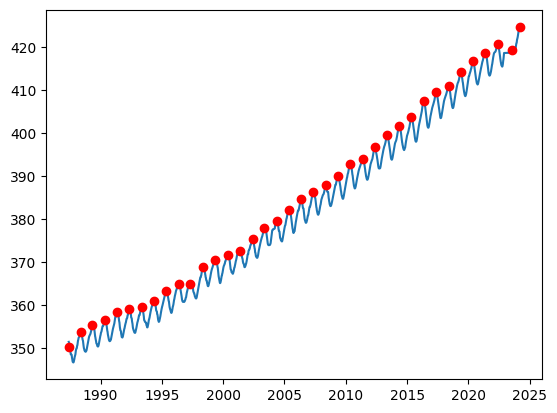
Step 6 - Computing statistics#
We will now extend the analysis. We will first write a function to extract the date and value of the dips in the data. We will then compute some statistics on the peaks and dips: the average year-on-year increase in the peak and dip values, the average time between peaks and dips, and the average difference between the peak and dip values.
First, write a function that uses NumPy to find the dips in the data, which you can call find_dips. The function should take a NumPy array as input and return a NumPy array of dip values and a NumPy array of dip indices. It should be very similar to the find_peaks function.
# SOLUTION
def find_dips(series):
peaks = (series[2:-2] < series[1:-3]) & (series[2:-2] < series[3:-1]) & (series[2:-2] < series[4:]) & (series[2:-2] < series[:-4])
indices = np.where(peaks == True)[0]
return series[indices], indices
# TEST
dips, dip_indices = find_dips(co2_concentration_smooth90_h)
plt.plot(decimal_year[45:-45],co2_concentration_smooth90_h[45:-45])
plt.plot(decimal_year[indices], peaks, 'ro')
plt.plot(decimal_year[dip_indices], dips, 'go')
plt.show()
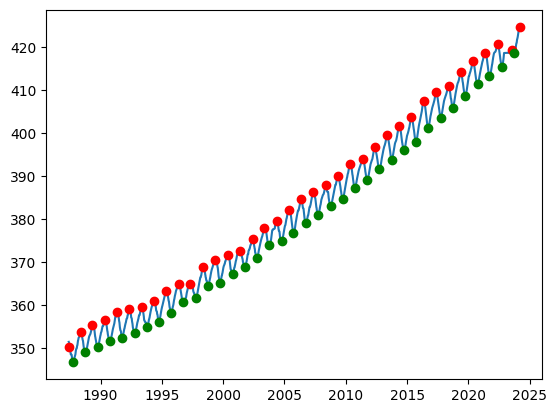
Now, we can write a function to compute the average year-on-year increase in the peak and dip values. The function should take a NumPy array of dip or peak values as input and return the average year-on-year increase. (You can assume that your list of dip and peak values has correctly identified the annual peak and dip.)
Call this function compute_average_difference and test it by running the test cell.
def compute_average_difference(series):
return np.mean(np.diff(series))
# TEST
# Note, first and last values are not included in the average as they are likely to be incorrect
average_peak_difference = compute_average_difference(peaks[1:-1])
average_dip_difference = compute_average_difference(dips[1:-1])
print(average_peak_difference, average_dip_difference)
1.8748410035709866 1.9514604054917082
The average increases should be close to 2. i.e. the CO2 concentration is increasing by about 2 parts per million per year.
Now write short pieces of code to find answers to the following questions.
Q1. What is the average difference between the annual peak and dip values?#
# SOLUTION
print(np.mean(peaks[:-1] - dips))
4.8954340161043195
You should have got an answer of about 5 parts per million.
Q2. What is the average time (in days) between a peak and the next dip?#
# SOLUTION
peak_indices = indices
delays = decimal_year[dip_indices[1:]] - decimal_year[peak_indices[1:-1]]
print(np.mean(delays) * 365)
136.10648148152495
You should get an answer of about 140 days.
Q3. What is the average time (in days) between a dip and the next peak?#
# SOLUTION
delays = decimal_year[peak_indices[1:-1]] - decimal_year[dip_indices[:-1]]
print(np.mean(delays) * 365)
228.89351851847508
You should get an answer of about 225 days.
Note that the average peak-to-dip and average dip-to-peak sums to about 365 days, that is, one year, but the dips are not exactly half way between the peaks. This is because the co2 concentration is increasing over time.
Q4. On which day of the year on average do the peaks and dips occurs?#
To compute this, you need to consider just the fractional part of the decimal year value of the peak and dip locations. You can retrieve this using the np.mod function.
# SOLUTION
peak_day = np.mean(np.mod(decimal_year[peak_indices[1:-1]], 1))* 365
dip_day = np.mean(np.mod(decimal_year[dip_indices[1:-1]], 1))* 365
print(peak_day, dip_day)
137.0393518518264 273.07142857144794
I calculate this to be day 135 (peak) and day 273 (dip), i.e., the peaks occur in mid-May and the dips occur at the end of September.
If you are interested in the reason for this, see
https://keelingcurve.ucsd.edu/2013/06/04/why-does-atmospheric-co2-peak-in-may/
Summary#
This lab class has provided a very short introduction to NumPy covering some basic methods for manipulating simple 1-dimensional data series. Later in the module we will be using it in more mathematical contexts for performing more complex operations involving higher dimensional arrays, e.g. 2-D matrix data. However, we will see that the basic ideas remain the same. We will be leveraging NumPy’s ability to perform operations on arrays very efficiently without the need for for-loops. This will allow us to write code that is more concise and easier to understand and which runs much faster than the equivalent code using only native Python data structures and for-loops.